Trello Android lately transformed from utilizing Gson to Moshi for dealing with JSON. It was a bit difficult so I needed to doc the method.
(For context, Trello Android primarily parses JSON. We hardly ever serialize JSON, and thus a lot of the focus right here is on deserializing.)
There have been three predominant causes for the change from Gson to Moshi: security, pace, and dangerous life selections.
Security – Gson doesn’t perceive Kotlin’s null security and can fortunately place null values into non-null properties. Additionally, default values solely typically work (relying on the constructor setup).
Pace – Loads of benchmarks (1, 2, 3) have demonstrated that Moshi is normally quicker than Gson. After we transformed, we arrange some benchmarks to see how real-world parsing in contrast in our app, and we noticed a 2x-3.5x speedup:
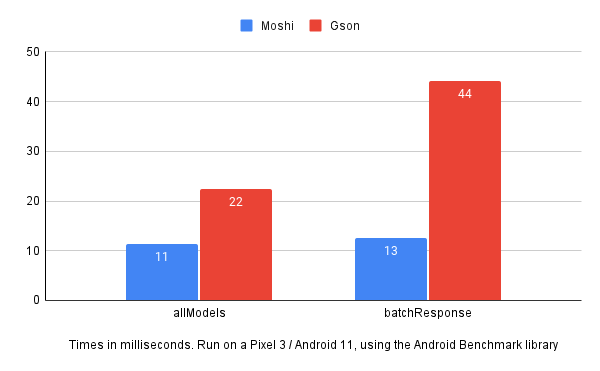
Dangerous life selections – As a substitute of utilizing Gson to parse JSON into easy fashions, we’d write elaborate, complicated, brittle customized deserializers that had fully an excessive amount of logic in them. Refactoring gave us a possibility to right this architectural snafu.
As for why we picked Moshi over opponents (e.g. Kotlin serialization), we typically belief Sq.’s libraries, we have used Moshi previously for tasks (each at work and at dwelling) and felt it labored properly. We didn’t do an in-depth research of alternate options.
Step one was to make sure that we may use function flags to modify between utilizing our previous Gson implementation and the brand new Moshi one. I wrote a JsonInterop
class which, based mostly on the flag, would both parse all JSON responses utilizing Gson or Moshi.
(I opted to keep away from utilizing instruments like moshi-gson-interop as a result of I needed to check whether or not Moshi parsing labored in its entirety. In the event you’d relatively have a mixture of Gson and Moshi on the identical time, that library could be helpful.)
Gson offers you alternatives to override the default naming of a key utilizing @SerializedName
. Moshi enables you to do the identical factor with @Json
. That is all properly and good, nevertheless it appeared very easy to me to make a mistake right here, the place a property is parsed beneath totally different names in Gson vs. Moshi.
Thus, I wrote some unit exams that may confirm that our generated Moshi adapters would have the identical end result as Gson’s parsing. Specifically, I examined…
- …that Moshi may generate an adapter (not essentially an accurate one!) for every class we needed to deserialize. (If it could not, Moshi would throw an exception.)
- …that every discipline annotated with
@SerializedName
was additionally annotated with@Json
(utilizing the identical key).
Between these two checks, it was simple to search out once I’d made a mistake updating our courses in later steps.
(I can’t embrace the supply right here, however principally we used Guava’s ClassPath to collect all our courses, then scan by way of them for issues.)
Gson lets you parse generic JSON timber utilizing JsonElement (and associates). We discovered this convenient in some contexts like parsing socket updates (the place we wouldn’t know the way, precisely, to parse the response mannequin till after some preliminary processing).
Clearly, Moshi is just not going to be pleased about utilizing Gson’s courses, so we switched to utilizing Map<String, Any?>
(and typically Record<Map<String, Any?>>
) for generic timber of information. Each Gson and Moshi can parse these:
enjoyable <T> fromJson(map: Map<String, Any?>?, clz: Class<T>): T? {
return if (USE_MOSHI) {
moshi.adapter(clz).fromJsonValue(map)
}
else {
gson.fromJson(gson.toJsonTree(map), clz)
}
}
As well as, Gson is pleasant in direction of parsing by way of Readers, however Moshi is just not. I discovered that utilizing BufferedSource was a very good different, as it may be transformed to a Reader for previous Gson code.
The best adapters for Moshi are those the place you simply slap @JsonClass
on them and name it a day. Sadly, as I discussed earlier, we had loads of unlucky customized deserialization logic in our Gson parser.
It’s fairly simple to write a customized Moshi adapter, however as a result of there was a lot customized logic in our deserializers, simply writing a single adapter wouldn’t reduce it. We ended up having to create interstitial fashions to parse the uncooked JSON, then adapt from that to the fashions we’re used to utilizing.
To offer a concrete instance, think about we have now a knowledge class Foo(val depend: Int)
, however the precise JSON we get again is of the shape:
{
"knowledge": {
"depend": 5
}
}
With Gson, we may simply manually take a look at the tree and seize the depend out of the knowledge
object, however we have now found that manner lies insanity. We might relatively simply parse utilizing easy POJOs, however we nonetheless need to output a Foo in the long run (so we do not have to alter our entire codebase).
To resolve that drawback, we’d create new fashions and use these in customized adapter, like so:
@JsonClass(generateAdapter = true) knowledge class JsonFoo(val knowledge: JsonData)
@JsonClass(generateAdapter = true) knowledge class JsonData(val depend: Int)
object FooAdapter {
@FromJson
enjoyable fromJson(json: JsonFoo): Foo {
return Foo(depend = json.knowledge.depend)
}
}
Voila! Now the parser can nonetheless output Foo, however we’re utilizing easy POJOs to mannequin our knowledge. It’s each simpler to interpret and simple to check.
Bear in mind how I stated that Gson will fortunately parse null values into non-null fashions? It seems that we have been (sadly) counting on this conduct in all types of locations. Specifically, Trello’s sockets typically return partial fashions – so whereas we’d usually anticipate, say, a card to come back again with a reputation, in some circumstances it received’t.
That meant having to watch our crashes for circumstances the place the Moshi would blow up (on account of a null worth) when Gson could be pleased as a clam. That is the place function flags actually shine, because you don’t need to must push a buggy parser on unsuspecting manufacturing customers!
After fixing a dozen of those bugs, I really feel like I’ve gained a hearty appreciation for non-JSON applied sciences with well-defined schemas like protocol buffers. There are loads of bugs I bumped into that merely wouldn’t have occurred if we had a contract between the server and the shopper.