Introduction
Python’s Counter is a sturdy knowledge construction conveniently counts parts in an iterable. It’s a part of the collections module and provides varied functionalities for counting, combining, and manipulating knowledge. On this article, we are going to discover the fundamentals of Counters, on a regular basis use instances, superior strategies, and ideas for optimizing efficiency utilizing Python’s Counter successfully.
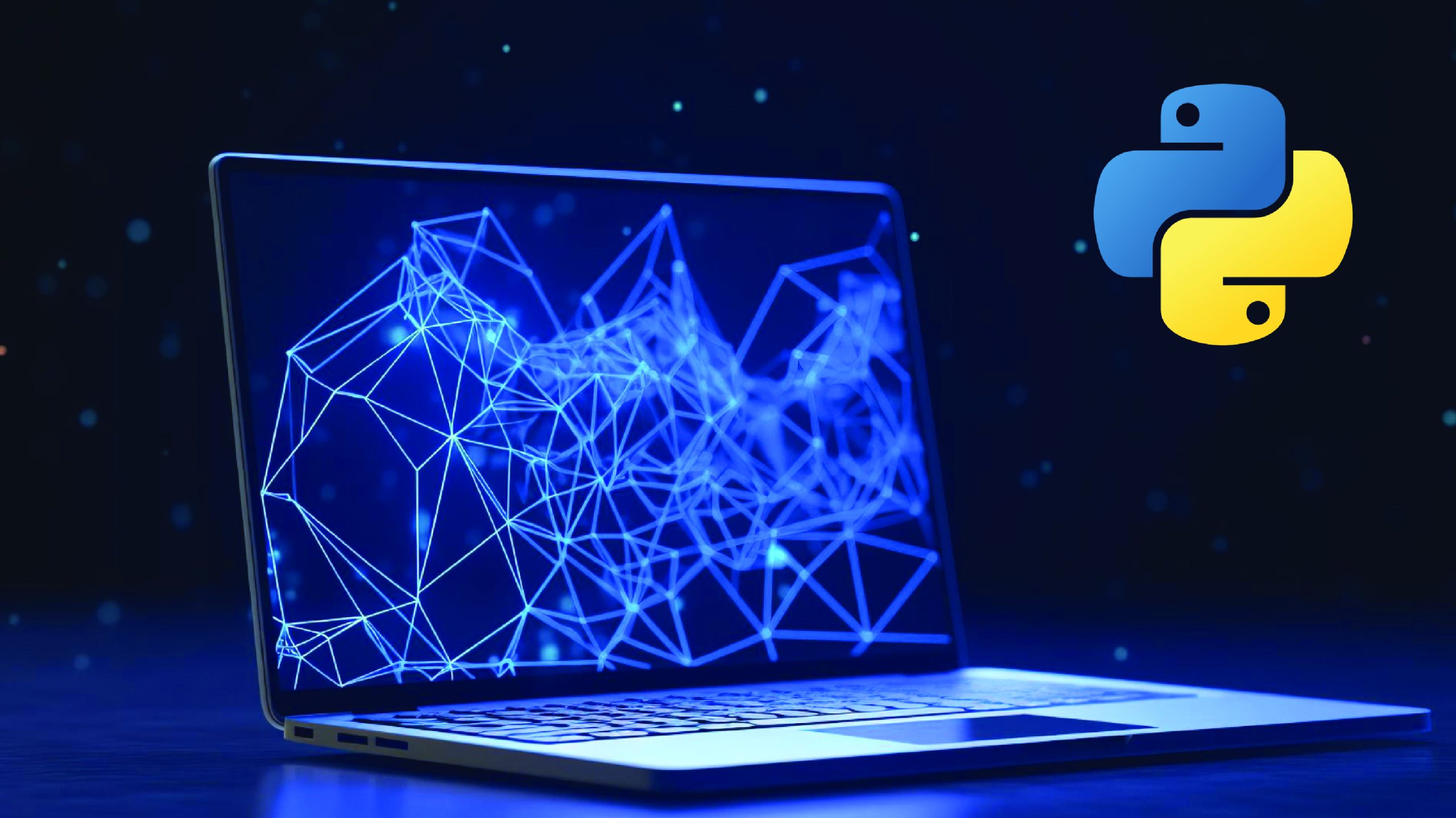
Additionally Learn: Python Enumerate(): Simplify Looping With Counters
Understanding the Fundamentals of Counters
Making a Counter Object
To create a Counter object, we are able to merely move an iterable to the Counter() constructor. The iterable is usually a checklist, tuple, string, or every other sequence. For instance:
from collections import Counter
my_list = [1, 2, 3, 1, 2, 3, 4, 5, 1, 2]
counter = Counter(my_list)
print(counter)
Output:
Counter({1: 3, 2: 3, 3: 2, 4: 1, 5: 1}
Accessing and Modifying Counter Components
We will entry the depend of a particular factor in a Counter utilizing the sq. bracket notation. Moreover, we are able to modify the depend of a component by assigning a brand new worth to it. For instance:
counter = Counter({'a': 3, 'b': 2, 'c': 1})
print(counter['a']) # Output: 3
counter['b'] = 5
print(counter) # Output: Counter({'a': 3, 'b': 5, 'c': 1})
Counting Components in an Iterable
Counters are notably helpful for counting the frequency of parts in an iterable. We will use the Counter’s most_common() technique to get an inventory of parts and their counts, sorted by the depend in descending order. For instance:
textual content = "Lorem ipsum dolor sit amet, consectetur adipiscing elit."
counter = Counter(textual content.decrease().break up())
print(counter.most_common(3))
Output:
[(‘ipsum’, 1), (‘lorem’, 1), (‘dolor’, 1)]
Combining Counters
We will mix a number of Counters utilizing the addition operator (+). This operation sums the counts of widespread parts in each Counters. For instance:
counter1 = Counter({'a': 3, 'b': 2, 'c': 1})
counter2 = Counter({'b': 4, 'c': 2, 'd': 1})
combined_counter = counter1 + counter2
print(combined_counter)
Output:
Counter({‘b’: 6, ‘a’: 3, ‘c’: 3, ‘d’: 1})
Eradicating Components from Counters
To take away parts from a Counter, we are able to use the del key phrase adopted by the factor we need to delete. This operation fully removes the factor from the Counter. For instance:
counter = Counter({'a': 3, 'b': 2, 'c': 1})
del counter['b']
print(counter)
Output:
Counter({‘a’: 3, ‘c’: 1})
Frequent Use Circumstances for Python’s Counter
Discovering Most Frequent Components
Counters may discover the commonest parts in any iterable. The most_common() technique returns an inventory of parts and their counts, sorted by the depend in descending order. For instance:
my_list = [1, 2, 3, 1, 2, 3, 4, 5, 1, 2]
counter = Counter(my_list)
print(counter.most_common(2))
Output:
[(1, 3), (2, 3)]
Figuring out Duplicate Components
Counters will help establish duplicate parts in an iterable by checking if the depend of any factor is bigger than 1. This may be helpful in knowledge cleansing and deduplication duties. For instance:
my_list = [1, 2, 3, 1, 2, 3, 4, 5, 1, 2]
counter = Counter(my_list)
duplicates = [element for element, count in counter.items() if count > 1]
print(duplicates)
Output:
[1, 2, 3]
Implementing Multisets and Baggage
Counters can be utilized to implement multisets and baggage, that are collections that permit duplicate parts. By treating the weather as keys and their counts as values, we are able to carry out varied operations on multisets and baggage effectively. For instance:
multiset = Counter({'a': 3, 'b': 2, 'c': 1})
print(multiset['a']) # Output: 3
bag = Counter({'a': 3, 'b': 2, 'c': 1})
print(bag['a']) # Output: 3
Monitoring Stock and Inventory Ranges
Counters can observe stock and inventory ranges in a retail or warehouse administration system. We will simply replace and retrieve the inventory ranges by associating every merchandise with its depend. For instance:
stock = Counter(apples=10, oranges=5, bananas=3)
print(stock['apples']) # Output: 10
stock['apples'] -= 2
print(stock['apples']) # Output: 8
Superior Strategies with Python’s Counter
Subtraction and Intersection of Counters
Counters help subtraction and intersection operations. Subtracting one Counter from one other subtracts the counts of widespread parts, whereas intersecting two Counters retains the minimal depend of widespread parts. For instance:
counter1 = Counter({'a': 3, 'b': 2, 'c': 1})
counter2 = Counter({'b': 4, 'c': 2, 'd': 1})
subtracted_counter = counter1 - counter2
print(subtracted_counter) # Output: Counter({'a': 3})
intersected_counter = counter1 & counter2
print(intersected_counter) # Output: Counter({'b': 2, 'c': 1})
Updating Counters with Arithmetic Operations
Counters may be up to date utilizing arithmetic operations similar to addition, subtraction, multiplication, and division. These operations replace the counts of parts within the Counter primarily based on the corresponding operation. For instance:
counter = Counter({'a': 3, 'b': 2, 'c': 1})
counter += Counter({'b': 4, 'c': 2, 'd': 1})
print(counter) # Output: Counter({'a': 3, 'b': 6, 'c': 3, 'd': 1})
counter -= Counter({'b': 2, 'c': 1})
print(counter) # Output: Counter({'a': 3, 'b': 4, 'c': 2, 'd': 1})
Working with Nested Counters
Counters may be nested to characterize hierarchical knowledge constructions. This permits us to depend parts at completely different ranges of granularity. For instance, we are able to have a Counter of Counters to characterize the counts of parts in several classes. For instance:
classes = Counter({
'fruit': Counter({'apple': 3, 'orange': 2}),
'vegetable': Counter({'carrot': 5, 'broccoli': 3}),
})
print(classes['fruit']['apple']) # Output: 3
print(classes['vegetable']['carrot']) # Output: 5
Dealing with Giant Datasets with Counter
Counters are environment friendly for dealing with massive datasets as a result of their optimized implementation. They use a hashtable to retailer the counts, which permits for constant-time entry and modification. This makes Counters appropriate for duties similar to counting phrase frequencies in massive texts or analyzing large knowledge. For instance:
textual content = "Lorem ipsum dolor sit amet, consectetur adipiscing elit." * 1000000
counter = Counter(textual content.decrease().break up())
print(counter.most_common(3))
Customizing Counter Conduct
Python’s Counter supplies a number of strategies and capabilities to customise its conduct. For instance, we are able to use the weather() technique to retrieve an iterator over the weather within the Counter, or use the subtract() technique to subtract counts from one other Counter. Moreover, we are able to use the most_common() perform to get the commonest parts from any iterable. For instance:
counter = Counter({'a': 3, 'b': 2, 'c': 1})
parts = counter.parts()
print(checklist(parts)) # Output: ['a', 'a', 'a', 'b', 'b', 'c']
counter.subtract({'a': 2, 'b': 1})
print(counter) # Output: Counter({'a': 1, 'b': 1, 'c': 1})
my_list = [1, 2, 3, 1, 2, 3, 4, 5, 1, 2]
most_common_elements = Counter(my_list).most_common(2)
print(most_common_elements) # Output: [(1, 3), (2, 3)]
Ideas for Optimizing Efficiency with Python’s Counter
Effectively Counting Giant Datasets
When counting massive datasets, utilizing the Counter’s replace() technique is advisable as a substitute of making a brand new Counter object for every factor. This avoids pointless reminiscence allocation and improves efficiency. For instance:
counter = Counter()
knowledge = [1, 2, 3, 1, 2, 3, 4, 5, 1, 2]
for factor in knowledge:
counter.replace([element])
print(counter)
Selecting the Proper Knowledge Construction
Think about the necessities of your job and select the suitable knowledge construction accordingly. If you happen to solely have to depend parts, a Counter is an acceptable selection. Nevertheless, for those who want extra functionalities similar to sorting or indexing, chances are you’ll want to make use of different knowledge constructions like dictionaries or lists.
Using Counter Strategies and Features
Python’s Counter supplies varied strategies and capabilities that may assist optimize efficiency. For instance, the most_common() technique can be utilized to retrieve the commonest parts effectively, whereas the weather() technique can be utilized to iterate over the weather with out creating a brand new checklist.
Conclusion
Python’s Counter is a flexible knowledge construction that gives highly effective functionalities for counting, combining, and manipulating knowledge. By understanding the fundamentals of Counters, exploring widespread use instances, mastering superior strategies, optimizing efficiency, and following finest practices, you may leverage the total potential of Python’s Counter in your initiatives. Whether or not you must depend phrase frequencies, discover the commonest parts, implement multisets, or observe stock, Counters supply a handy and environment friendly answer. So begin utilizing Python’s Counter at present and unlock the facility of counting in your code.