Introduction
Arrays in Python are knowledge buildings that may maintain a number of values of the identical knowledge sort. They supply a approach to retailer and manipulate collections of knowledge effectively. On this article, we’ll discover how one can work with arrays in Python, together with creating arrays, accessing and manipulating array components, performing array operations, working with multi-dimensional arrays, and utilizing arrays in real-world functions.
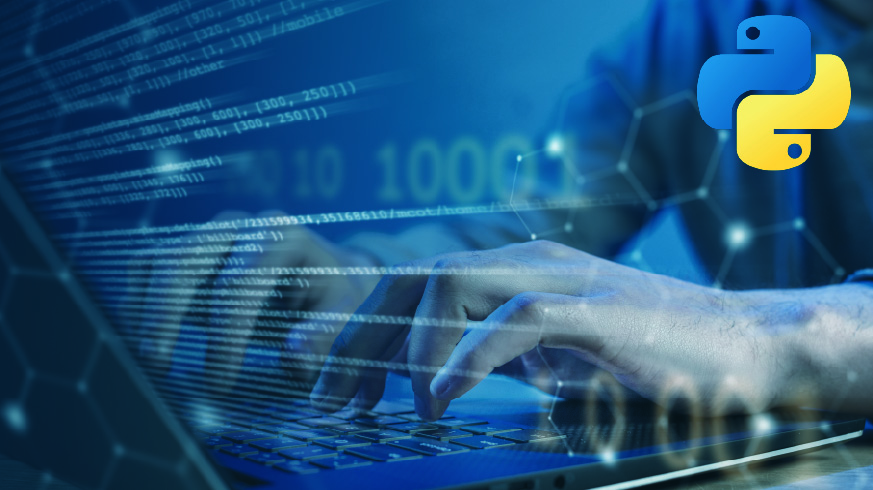
Creating Arrays in Python
There are a number of methods to create arrays in Python. One frequent methodology is utilizing the NumPy library. NumPy helps multi-dimensional arrays and numerous mathematical capabilities for array manipulation. Right here’s how one can create a NumPy array:
import numpy as np
my_array = np.array([1, 2, 3, 4, 5])
sort(my_array)
Output: numpy.ndarray
You can even create arrays utilizing the Array module in Python, which gives further functionalities for array manipulation.
import array
# Create an array of integers
int_array = array.array('i', [1, 2, 3, 4, 5])
sort(int_array)
Output: array.array
Accessing and Manipulating Arrays
After getting created an array, you possibly can entry and manipulate its components utilizing indexing and slicing. Indexing lets you entry particular components within the array, whereas slicing lets you extract a subset of components. Right here’s an instance:
my_array = np.array([1, 2, 3, 4, 5])
# Accessing components
print(my_array[0]) # Output: 1
# Slicing
print(my_array[1:4])
Output: [2, 3, 4]
You can even add and take away components from an array and replace present components to change the array’s content material.
# Including components
my_array = np.append(my_array, 6)
print("After including a component:", my_array) # Output: [1 2 3 4 5 6]
# Eradicating components
my_array = np.delete(my_array, 2) # Take away ingredient at index 2
print("After eradicating a component:", my_array) # Output: [1 2 4 5 6]
# Updating components
my_array[3] = 7
print("After updating a component:", my_array)
Output: [1 2 4 7 6]
Array Operations and Capabilities
Arrays in Python help numerous operations and capabilities for environment friendly knowledge manipulation. You may concatenate arrays, reshape their dimensions, type components, and seek for particular values inside an array. These operations are important for performing complicated knowledge processing duties.
# Array Concatenation
array1 = np.array([1, 2, 3])
array2 = np.array([4, 5, 6])
concatenated_array = np.concatenate((array1, array2))
print(concatenated_array) # Output: [1 2 3 4 5 6]
# Array Reshaping
reshaped_array = concatenated_array.reshape(2, 3)
print(reshaped_array)
Output: [[1 2 3]
[4 5 6]]
# Array Sorting
sorted_array = np.type(concatenated_array)
print(sorted_array) # Output: [1 2 3 4 5 6]
# Array Looking out
index = np.the place(sorted_array == 3)
print(index)
Output: (array([2]),)
Multi-dimensional Arrays
Along with one-dimensional arrays, Python additionally helps multi-dimensional arrays utilizing NumPy. Multi-dimensional arrays characterize complicated knowledge buildings corresponding to matrices and tensors. Right here’s an instance of making and accessing a multi-dimensional array:
multi_array = np.array([[1, 2, 3], [4, 5, 6]])
print(multi_array)
Output: [[1 2 3]
[4 5 6]]
You may carry out numerous operations on multi-dimensional arrays, corresponding to element-wise arithmetic operations, matrix multiplication, and transposition.
Additionally learn: Capabilities 101 – Introduction to Capabilities in Python For Absolute Inexperienced persons.
Comparability of Numpy Array Technique and Array Module
- NumPy Array Technique
- Gives intensive performance for numerical computing.
- Helps multidimensional arrays and various knowledge sorts.
- Optimized for environment friendly mathematical operations.
- Extensively utilized in scientific computing and knowledge evaluation.
- Supplies wealthy array manipulation and broadcasting.
- Most popular for complicated duties requiring superior functionalities.
- Array Module
- Supplies a less complicated various to primary array manipulation.
- Arrays are one-dimensional and homogeneous.
- Gives restricted knowledge sorts and primary operations.
- Extra reminiscence environment friendly in comparison with lists for giant datasets.
- Appropriate for simple duties with homogeneous knowledge.
- Lacks superior options and optimizations of NumPy arrays.
Working with Arrays in Actual-world Purposes
Arrays are essential in numerous real-world functions, together with knowledge evaluation and visualization, machine studying and AI, and scientific computing. They supply a basis for dealing with massive datasets, performing complicated calculations, and implementing algorithms effectively.
Greatest Practices for Utilizing Arrays in Python
When working with arrays in Python, it’s important to observe finest practices for environment friendly reminiscence administration, selecting the best knowledge construction, and optimizing array operations. By optimizing your code and knowledge buildings, you possibly can enhance efficiency and scalability in your functions.
Conclusion
In conclusion, arrays are highly effective knowledge buildings in Python that allow you to retailer, manipulate, and analyze knowledge collections successfully. By understanding how one can create arrays, entry and manipulate array components, carry out array operations, and work with multi-dimensional arrays, you possibly can leverage the complete potential of arrays in your Python initiatives.
By following finest practices and exploring the huge array of functionalities out there in Python libraries corresponding to NumPy, you possibly can simply take your array manipulation abilities to the following degree and sort out complicated knowledge processing duties. Begin experimenting with arrays in Python immediately and unlock a world of prospects in knowledge science, machine studying, and scientific computing.
In case you are searching for an internet Python course, take a look at this “Be taught Python for Information Science.”