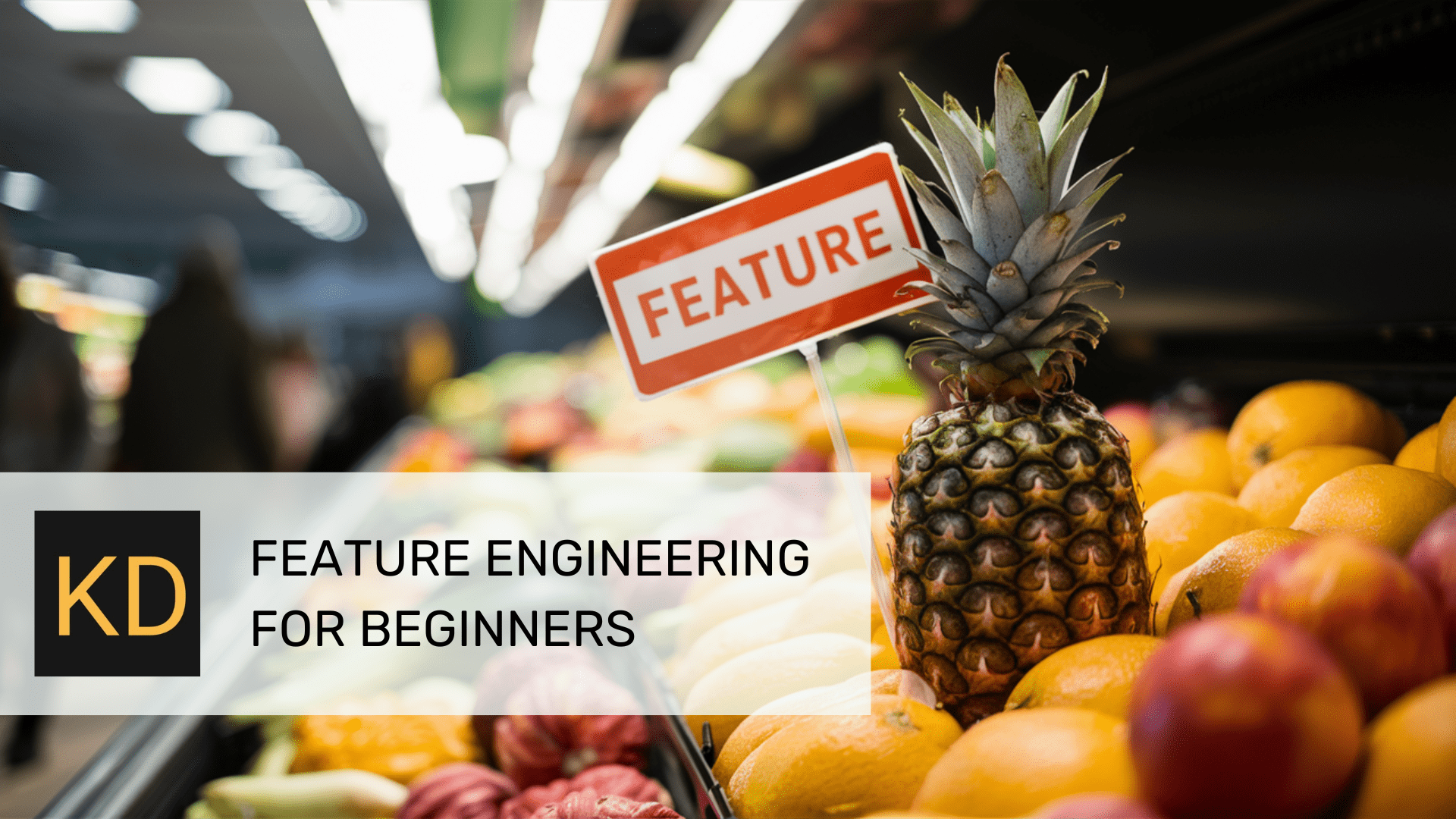
Picture created by Writer
Introduction
Characteristic engineering is likely one of the most essential features of the machine studying pipeline. It’s the apply of making and modifying options, or variables, for the needs of bettering mannequin efficiency. Properly-designed options can remodel weak fashions into sturdy ones, and it’s by way of characteristic engineering that fashions can turn out to be each extra sturdy and correct. Characteristic engineering acts because the bridge between the dataset and the mannequin, giving the mannequin the whole lot it must successfully remedy an issue.
This can be a information meant for brand new information scientists, information engineers, and machine studying practitioners. The target of this text is to speak basic characteristic engineering ideas and supply a toolbox of strategies that may be utilized to real-world situations. My intention is that, by the top of this text, you’ll be armed with sufficient working data about characteristic engineering to use it to your individual datasets to be fully-equipped to start creating highly effective machine studying fashions.
Understanding Options
Options are measurable traits of any phenomenon that we’re observing. They’re the granular parts that make up the info with which fashions function upon to make predictions. Examples of options can embrace issues like age, earnings, a timestamp, longitude, worth, and nearly the rest one can consider that may be measured or represented in some type.
There are completely different characteristic sorts, the principle ones being:
- Numerical Options: Steady or discrete numeric sorts (e.g. age, wage)
- Categorical Options: Qualitative values representing classes (e.g. gender, shoe measurement sort)
- Textual content Options: Phrases or strings of phrases (e.g. “this” or “that” or “even this”)
- Time Sequence Options: Information that’s ordered by time (e.g. inventory costs)
Options are essential in machine studying as a result of they immediately affect a mannequin’s potential to make predictions. Properly-constructed options enhance mannequin efficiency, whereas dangerous options make it tougher for a mannequin to provide sturdy predictions. Characteristic choice and have engineering are preprocessing steps within the machine studying course of which can be used to organize the info to be used by studying algorithms.
A distinction is made between characteristic choice and have engineering, although each are essential in their very own proper:
- Characteristic Choice: The culling of essential options from the whole set of all obtainable options, thus decreasing dimensionality and selling mannequin efficiency
- Characteristic Engineering: The creation of latest options and subsequent altering of current ones, all in the help of making a mannequin carry out higher
By deciding on solely an important options, characteristic choice helps to solely depart behind the sign within the information, whereas characteristic engineering creates new options that assist to mannequin the result higher.
Fundamental Strategies in Characteristic Engineering
Whereas there are a variety of fundamental characteristic engineering strategies at our disposal, we’ll stroll by way of a number of the extra essential and well-used of those.
Dealing with Lacking Values
It’s common for datasets to comprise lacking data. This may be detrimental to a mannequin’s efficiency, which is why you will need to implement methods for coping with lacking information. There are a handful of frequent strategies for rectifying this subject:
- Imply/Median Imputation: Filling lacking areas in a dataset with the imply or median of the column
- Mode Imputation: Filling lacking spots in a dataset with the commonest entry in the identical column
- Interpolation: Filling in lacking information with values of information factors round it
These fill-in strategies needs to be utilized based mostly on the character of the info and the potential impact that the strategy might need on the top mannequin.
Coping with lacking data is essential in protecting the integrity of the dataset in tact. Right here is an instance Python code snippet that demonstrates numerous information filling strategies utilizing the pandas
library.
import pandas as pd
from sklearn.impute import SimpleImputer
# Pattern DataFrame
information = {'age': [25, 30, np.nan, 35, 40], 'wage': [50000, 60000, 55000, np.nan, 65000]}
df = pd.DataFrame(information)
# Fill in lacking ages utilizing the imply
mean_imputer = SimpleImputer(technique='imply')
df['age'] = mean_imputer.fit_transform(df[['age']])
# Fill within the lacking salaries utilizing the median
median_imputer = SimpleImputer(technique='median')
df['salary'] = median_imputer.fit_transform(df[['salary']])
print(df)
Encoding of Categorical Variables
Recalling that the majority machine studying algorithms are greatest (or solely) geared up to take care of numeric information, categorical variables should usually be mapped to numerical values to ensure that stated algorithms to higher interpret them. The commonest encoding schemes are the next:
- One-Sizzling Encoding: Producing separate columns for every class
- Label Encoding: Assigning an integer to every class
- Goal Encoding: Encoding classes by their particular person end result variable averages
The encoding of categorical information is important for planting the seeds of understanding in lots of machine studying fashions. The correct encoding methodology is one thing you’ll choose based mostly on the precise state of affairs, together with each the algorithm at use and the dataset.
Beneath is an instance Python script for the encoding of categorical options utilizing pandas
and parts of scikit-learn
.
import pandas as pd
from sklearn.preprocessing import OneHotEncoder, LabelEncoder
# Pattern DataFrame
information = {'coloration': ['red', 'blue', 'green', 'blue', 'red']}
df = pd.DataFrame(information)
# Implementing one-hot encoding
one_hot_encoder = OneHotEncoder()
one_hot_encoding = one_hot_encoder.fit_transform(df[['color']]).toarray()
df_one_hot = pd.DataFrame(one_hot_encoding, columns=one_hot_encoder.get_feature_names(['color']))
# Implementing label encoding
label_encoder = LabelEncoder()
df['color_label'] = label_encoder.fit_transform(df['color'])
print(df)
print(df_one_hot)
Scaling and Normalizing Information
For good efficiency of many machine studying strategies, scaling and normalization must be carried out in your information. There are a number of strategies for scaling and normalizing information, comparable to:
- Standardization: Remodeling information in order that it has a imply of 0 and a typical deviation of 1
- Min-Max Scaling: Scaling information to a set vary, comparable to [0, 1]
- Strong Scaling: Scaling excessive and low values iteratively by the median and interquartile vary, respectively
The scaling and normalization of information is essential for making certain that characteristic contributions are equitable. These strategies enable the various characteristic values to contribute to a mannequin commensurately.
Beneath is an implementation, utilizing scikit-learn
, that exhibits the way to full information that has been scaled and normalized.
import pandas as pd
from sklearn.preprocessing import StandardScaler, MinMaxScaler, RobustScaler
# Pattern DataFrame
information = {'age': [25, 30, 35, 40, 45], 'wage': [50000, 60000, 55000, 65000, 70000]}
df = pd.DataFrame(information)
# Standardize information
scaler_standard = StandardScaler()
df['age_standard'] = scaler_standard.fit_transform(df[['age']])
# Min-Max Scaling
scaler_minmax = MinMaxScaler()
df['salary_minmax'] = scaler_minmax.fit_transform(df[['salary']])
# Strong Scaling
scaler_robust = RobustScaler()
df['salary_robust'] = scaler_robust.fit_transform(df[['salary']])
print(df)
The fundamental strategies above together with the corresponding instance code present pragmatic options for lacking information, encoding categorical variables, and scaling and normalizing information utilizing powerhouse Python instruments pandas
and scikit-learn
. These strategies could be built-in into your individual characteristic engineering course of to enhance your machine studying fashions.
Superior Strategies in Characteristic Engineering
We now flip our consideration to to extra superior featured engineering strategies, and embrace some pattern Python code for implementing these ideas.
Characteristic Creation
With characteristic creation, new options are generated or modified to style a mannequin with higher efficiency. Some strategies for creating new options embrace:
- Polynomial Options: Creation of higher-order options with current options to seize extra advanced relationships
- Interplay Phrases: Options generated by combining a number of options to derive interactions between them
- Area-Particular Characteristic Technology: Options designed based mostly on the intricacies of topics throughout the given downside realm
Creating new options with tailored that means can significantly assist to spice up mannequin efficiency. The subsequent script showcases how characteristic engineering can be utilized to carry latent relationships in information to mild.
import pandas as pd
import numpy as np
# Pattern DataFrame
information = {'x1': [1, 2, 3, 4, 5], 'x2': [10, 20, 30, 40, 50]}
df = pd.DataFrame(information)
# Polynomial Options
df['x1_squared'] = df['x1'] ** 2
df['x1_x2_interaction'] = df['x1'] * df['x2']
print(df)
Dimensionality Discount
So as to simplify fashions and enhance their efficiency, it may be helpful to downsize the variety of mannequin options. Dimensionality discount strategies that may assist obtain this purpose embrace:
- PCA (Principal Part Evaluation): Transformation of predictors into a brand new characteristic set comprised of linearly impartial mannequin options
- t-SNE (t-Distributed Stochastic Neighbor Embedding): Dimension discount that’s used for visualization functions
- LDA (Linear Discriminant Evaluation): Discovering new mixtures of mannequin options which can be efficient for deconstructing completely different courses
So as to shrink the dimensions of your dataset and keep its relevancy, dimensional discount strategies will assist. These strategies had been devised to deal with the high-dimensional points associated to information, comparable to overfitting and computational demand.
An illustration of information shrinking applied with scikit-learn
is proven subsequent.
import pandas as pd
from sklearn.decomposition import PCA
# Pattern DataFrame
information = {'feature1': [2.5, 0.5, 2.2, 1.9, 3.1], 'feature2': [2.4, 0.7, 2.9, 2.2, 3.0]}
df = pd.DataFrame(information)
# Use PCA for Dimensionality Discount
pca = PCA(n_components=1)
df_pca = pca.fit_transform(df)
df_pca = pd.DataFrame(df_pca, columns=['principal_component'])
print(df_pca)
Time Sequence Characteristic Engineering
With time-based datasets, particular characteristic engineering strategies have to be used, comparable to:
- Lag Options: Former information factors are used to derive mannequin predictive options
- Rolling Statistics: Information statistics are calculated throughout information home windows, comparable to rolling means
- Seasonal Decomposition: Information is partitioned into sign, pattern, and random noise classes
Temporal fashions want various augmentation in comparison with direct mannequin becoming. These strategies comply with temporal dependence and patterns to make the predictive mannequin sharper.
An illustration of time sequence options augmenting utilized utilizing pandas
is proven subsequent as effectively.
import pandas as pd
import numpy as np
# Pattern DataFrame
date_rng = pd.date_range(begin="1/1/2022", finish='1/10/2022', freq='D')
information = {'date': date_rng, 'worth': [100, 110, 105, 115, 120, 125, 130, 135, 140, 145]}
df = pd.DataFrame(information)
df.set_index('date', inplace=True)
# Lag Options
df['value_lag1'] = df['value'].shift(1)
# Rolling Statistics
df['value_rolling_mean'] = df['value'].rolling(window=3).imply()
print(df)
The above examples display sensible purposes of superior characteristic engineering strategies, by way of utilization of pandas
and scikit-learn
. By using these strategies you possibly can improve the predictive energy of your mannequin.
Sensible Suggestions and Greatest Practices
Listed below are just a few easy however essential suggestions to remember whereas working by way of your characteristic engineering course of.
- Iteration: Characteristic engineering is a trial-and-error course of, and you’re going to get higher with it every time you iterate. Take a look at completely different characteristic engineering concepts to seek out one of the best set of options.
- Area Information: Make the most of experience from those that know the subject material effectively when creating options. Generally refined relationships could be captured with realm-specific data.
- Validation and Understanding of Options: By understanding which options are most essential to your mode, you might be geared up to make essential choices. Instruments for figuring out characteristic significance embrace:
- SHAP (SHapley Additive exPlanations): Serving to to quantify the contribution of every characteristic in predictions
- LIME (Native Interpretable Mannequin-agnostic Explanations): Showcasing the that means of mannequin predictions in plain language
An optimum mixture of complexity and interpretability is important for having each good and easy to digest outcomes.
Conclusion
This brief information has addressed basic characteristic engineering ideas, in addition to fundamental and superior strategies, and sensible suggestions and greatest practices. What many would contemplate a number of the most essential characteristic engineering practices — coping with lacking data, encoding of categorical information, scaling information, and creation of latest options — had been lined.
Characteristic engineering is a apply that turns into higher with execution, and I hope you might have been in a position to take one thing away with you which will enhance your information science abilities. I encourage you to use these strategies to your individual work and to study out of your experiences.
Keep in mind that, whereas the precise share varies relying on who tells it, a majority of any machine studying mission is spent within the information preparation and preprocessing part. Characteristic engineering is part of this prolonged part, and as such needs to be seen with the import that it calls for. Studying to see characteristic engineering what it’s — a serving to hand within the modeling course of — ought to make it extra digestible to newcomers.
Pleased engineering!
Matthew Mayo (@mattmayo13) holds a Grasp’s diploma in laptop science and a graduate diploma in information mining. As Managing Editor, Matthew goals to make advanced information science ideas accessible. His skilled pursuits embrace pure language processing, machine studying algorithms, and exploring rising AI. He’s pushed by a mission to democratize data within the information science neighborhood. Matthew has been coding since he was 6 years previous.