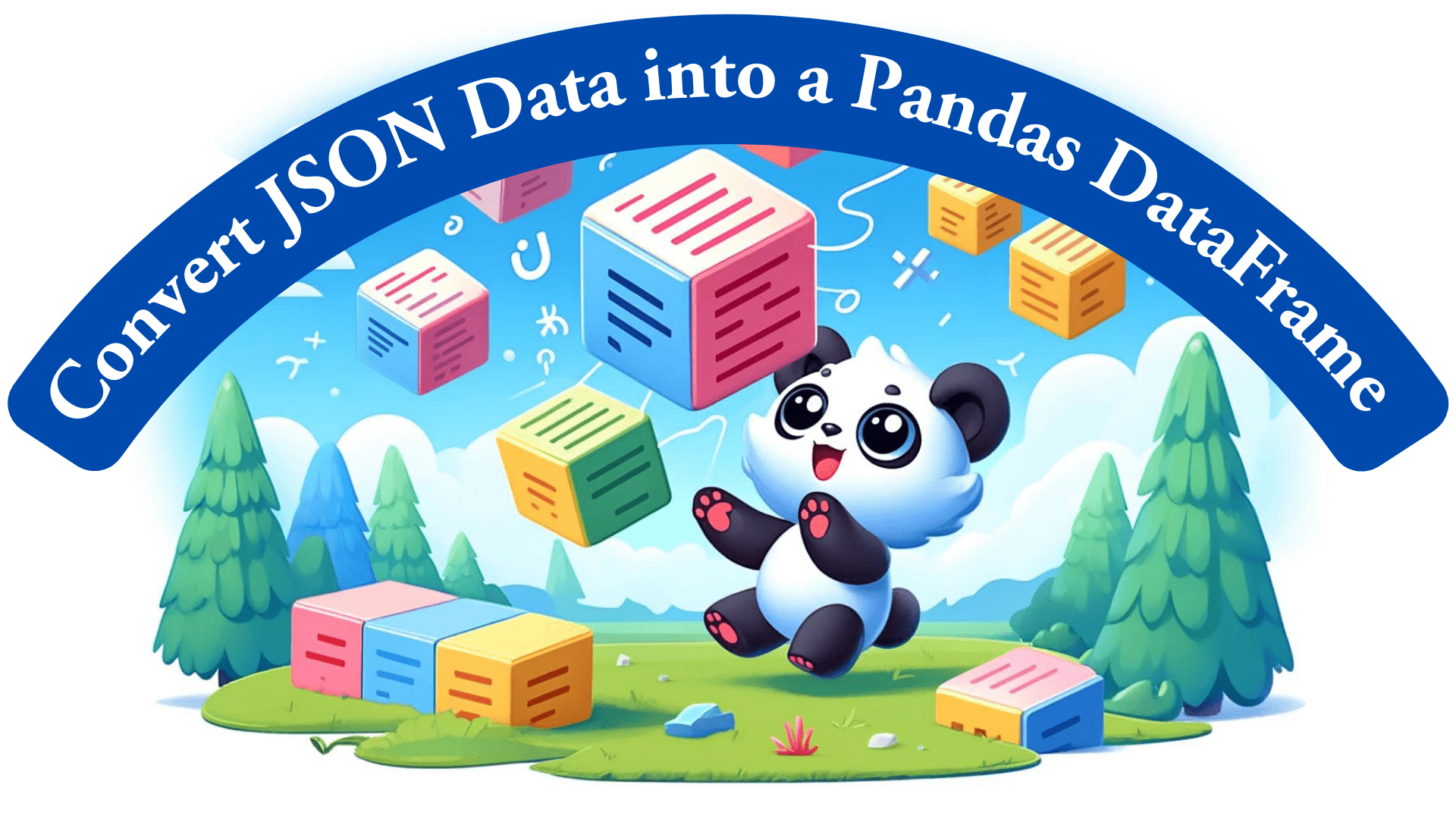
Picture by Creator | DALLE-3 & Canva
Â
For those who’ve ever had the prospect to work with information, you’ve got most likely come throughout the necessity to load JSON information (quick for JavaScript Object Notation) right into a Pandas DataFrame for additional evaluation. JSON information retailer information in a format that’s clear for individuals to learn and in addition easy for computer systems to know. Nevertheless, JSON information can generally be sophisticated to navigate by way of. Subsequently, we load them right into a extra structured format like DataFrames – that’s arrange like a spreadsheet with rows and columns.
I’ll present you two other ways to transform JSON information right into a Pandas DataFrame. Earlier than we talk about these strategies, let’s suppose this dummy nested JSON file that I am going to use for example all through this text.
{
"books": [
{
"title": "One Hundred Years of Solitude",
"author": "Gabriel Garcia Marquez",
"reviews": [
{
"reviewer": {
"name": "Kanwal Mehreen",
"location": "Islamabad, Pakistan"
},
"rating": 4.5,
"comments": "Magical and completely breathtaking!"
},
{
"reviewer": {
"name": "Isabella Martinez",
"location": "Bogotá, Colombia"
},
"rating": 4.7,
"comments": "A marvelous journey through a world of magic."
}
]
},
{
"title": "Issues Fall Aside",
"writer": "Chinua Achebe",
"critiques": [
{
"reviewer": {
"name": "Zara Khan",
"location": "Lagos, Nigeria"
},
"rating": 4.9,
"comments": "Things Fall Apart is the best of contemporary African literature."
}]}]}
Â
The above-mentioned JSON information represents a listing of books, the place every ebook has a title, writer, and a listing of critiques. Every evaluate, in flip, has a reviewer (with a reputation and placement) and a ranking and feedback.
Â
Methodology 1: Utilizing the json.load()
and pd.DataFrame()
capabilities
Â
The simplest and most simple strategy is to make use of the built-in json.load()
operate to parse our JSON information. This can convert it right into a Python dictionary, and we are able to then create the DataFrame straight from the ensuing Python information construction. Nevertheless, it has an issue – it will probably solely deal with single nested information. So, for the above case, in case you solely use these steps with this code:
import json
import pandas as pd
#Load the JSON information
with open('books.json','r') as f:
information = json.load(f)
#Create a DataFrame from the JSON information
df = pd.DataFrame(information['books'])
df
Â
Your output may appear to be this:
Output:
Â
Â
Within the critiques column, you may see the complete dictionary. Subsequently, if you would like the output to seem accurately, it’s a must to manually deal with the nested construction. This may be executed as follows:
#Create a DataFrame from the nested JSON information
df = pd.DataFrame([
{
'title': book['title'],
'writer': ebook['author'],
'reviewer_name': evaluate['reviewer']['name'],
'reviewer_location': evaluate['reviewer']['location'],
'ranking': evaluate['rating'],
'feedback': evaluate['comments']
}
for ebook in information['books']
for evaluate in ebook['reviews']
])
Â
Up to date Output:
Â
Â
Right here, we’re utilizing record comprehension to create a flat record of dictionaries, the place every dictionary comprises the ebook data and the corresponding evaluate. We then create the Pandas DataFrae utilizing this.
Nevertheless the problem with this strategy is that it calls for extra handbook effort to handle the nested construction of the JSON information. So, what now? Do we’ve got every other choice?
Completely! I imply, come on. On condition that we’re within the twenty first century, dealing with such an issue with no answer appears unrealistic. Let’s examine the opposite strategy.
Â
Methodology 2 (Really helpful): Utilizing the json_normalize()
operate
Â
The json_normalize()
operate from the Pandas library is a greater approach to handle nested JSON information. It robotically flattens the nested construction of the JSON information, making a DataFrame from the ensuing information. Let’s check out the code:
import pandas as pd
import json
#Load the JSON information
with open('books.json', 'r') as f:
information = json.load(f)
#Create the DataFrame utilizing json_normalize()
df = pd.json_normalize(
information=information['books'],
meta=['title', 'author'],
record_path="critiques",
errors="elevate"
)
df
Â
Output:
Â
Â
The json_normalize()
operate takes the next parameters:
- information: The enter information, which generally is a record of dictionaries or a single dictionary. On this case, it is the info dictionary loaded from the JSON file.
- record_path: The trail within the JSON information to the data you need to normalize. On this case, it is the ‘critiques’ key.
- meta: Extra fields to incorporate within the normalized output from the JSON doc. On this case, we’re utilizing the ‘title’ and ‘writer’ fields. Word that columns in metadata often seem on the finish. That is how this operate works. So far as the evaluation is worried, it would not matter, however for some magical cause, you need these columns to seem earlier than. Sorry, however it’s a must to do them manually.
- errors: The error dealing with technique, which may be ‘ignore’, ‘elevate’, or ‘warn’. We’ve set it to ‘elevate’, so if there are any errors in the course of the normalization course of, it’ll elevate an exception.
Â
Wrapping Up
Â
Each of those strategies have their very own benefits and use circumstances, and the selection of technique will depend on the construction and complexity of the JSON information. If the JSON information has a really nested construction, the json_normalize()
operate is perhaps the most suitable choice, as it will probably deal with the nested information robotically. If the JSON information is comparatively easy and flat, the pd.read_json()
operate is perhaps the simplest and most simple strategy.
When coping with giant JSON information, it is essential to consider reminiscence utilization and efficiency since loading the entire file into reminiscence won’t work. So, you might need to look into different choices like streaming the info, lazy loading, or utilizing a extra memory-efficient format like Parquet.
Â
Â
Kanwal Mehreen Kanwal is a machine studying engineer and a technical author with a profound ardour for information science and the intersection of AI with medication. She co-authored the e book “Maximizing Productiveness with ChatGPT”. As a Google Era Scholar 2022 for APAC, she champions range and educational excellence. She’s additionally acknowledged as a Teradata Variety in Tech Scholar, Mitacs Globalink Analysis Scholar, and Harvard WeCode Scholar. Kanwal is an ardent advocate for change, having based FEMCodes to empower ladies in STEM fields.