At present, most functions can ship a whole bunch of requests for a single web page.
For instance, my Twitter dwelling web page sends round 300 requests, and an Amazon
product particulars web page sends round 600 requests. A few of them are for static
belongings (JavaScript, CSS, font recordsdata, icons, and so forth.), however there are nonetheless
round 100 requests for async information fetching – both for timelines, mates,
or product suggestions, in addition to analytics occasions. That’s fairly a
lot.
The primary cause a web page could include so many requests is to enhance
efficiency and consumer expertise, particularly to make the applying really feel
quicker to the top customers. The period of clean pages taking 5 seconds to load is
lengthy gone. In fashionable internet functions, customers sometimes see a primary web page with
model and different components in lower than a second, with extra items
loading progressively.
Take the Amazon product element web page for instance. The navigation and high
bar seem virtually instantly, adopted by the product pictures, transient, and
descriptions. Then, as you scroll, “Sponsored” content material, rankings,
suggestions, view histories, and extra seem.Usually, a consumer solely desires a
fast look or to check merchandise (and verify availability), making
sections like “Clients who purchased this merchandise additionally purchased” much less important and
appropriate for loading through separate requests.
Breaking down the content material into smaller items and loading them in
parallel is an efficient technique, nevertheless it’s removed from sufficient in massive
functions. There are lots of different facets to think about in relation to
fetch information accurately and effectively. Knowledge fetching is a chellenging, not
solely as a result of the character of async programming would not match our linear mindset,
and there are such a lot of elements could cause a community name to fail, but in addition
there are too many not-obvious circumstances to think about below the hood (information
format, safety, cache, token expiry, and so forth.).
On this article, I wish to talk about some widespread issues and
patterns it is best to take into account in relation to fetching information in your frontend
functions.
We’ll start with the Asynchronous State Handler sample, which decouples
information fetching from the UI, streamlining your utility structure. Subsequent,
we’ll delve into Fallback Markup, enhancing the intuitiveness of your information
fetching logic. To speed up the preliminary information loading course of, we’ll
discover methods for avoiding Request
Waterfall and implementing Parallel Knowledge Fetching. Our dialogue will then cowl Code Splitting to defer
loading non-critical utility elements and Prefetching information based mostly on consumer
interactions to raise the consumer expertise.
I imagine discussing these ideas by way of an easy instance is
the perfect strategy. I goal to start out merely after which introduce extra complexity
in a manageable manner. I additionally plan to maintain code snippets, notably for
styling (I am using TailwindCSS for the UI, which may end up in prolonged
snippets in a React part), to a minimal. For these within the
full particulars, I’ve made them obtainable on this
repository.
Developments are additionally taking place on the server aspect, with methods like
Streaming Server-Aspect Rendering and Server Parts gaining traction in
numerous frameworks. Moreover, numerous experimental strategies are
rising. Nonetheless, these matters, whereas doubtlessly simply as essential, is likely to be
explored in a future article. For now, this dialogue will focus
solely on front-end information fetching patterns.
It is necessary to notice that the methods we’re protecting aren’t
unique to React or any particular frontend framework or library. I’ve
chosen React for illustration functions on account of my intensive expertise with
it in recent times. Nonetheless, ideas like Code Splitting,
Prefetching are
relevant throughout frameworks like Angular or Vue.js. The examples I am going to share
are widespread eventualities you may encounter in frontend improvement, regardless
of the framework you utilize.
That mentioned, let’s dive into the instance we’re going to make use of all through the
article, a Profile
display of a Single-Web page Software. It is a typical
utility you might need used earlier than, or no less than the state of affairs is typical.
We have to fetch information from server aspect after which at frontend to construct the UI
dynamically with JavaScript.
Introducing the applying
To start with, on Profile
we’ll present the consumer’s transient (together with
identify, avatar, and a brief description), after which we additionally wish to present
their connections (much like followers on Twitter or LinkedIn
connections). We’ll must fetch consumer and their connections information from
distant service, after which assembling these information with UI on the display.
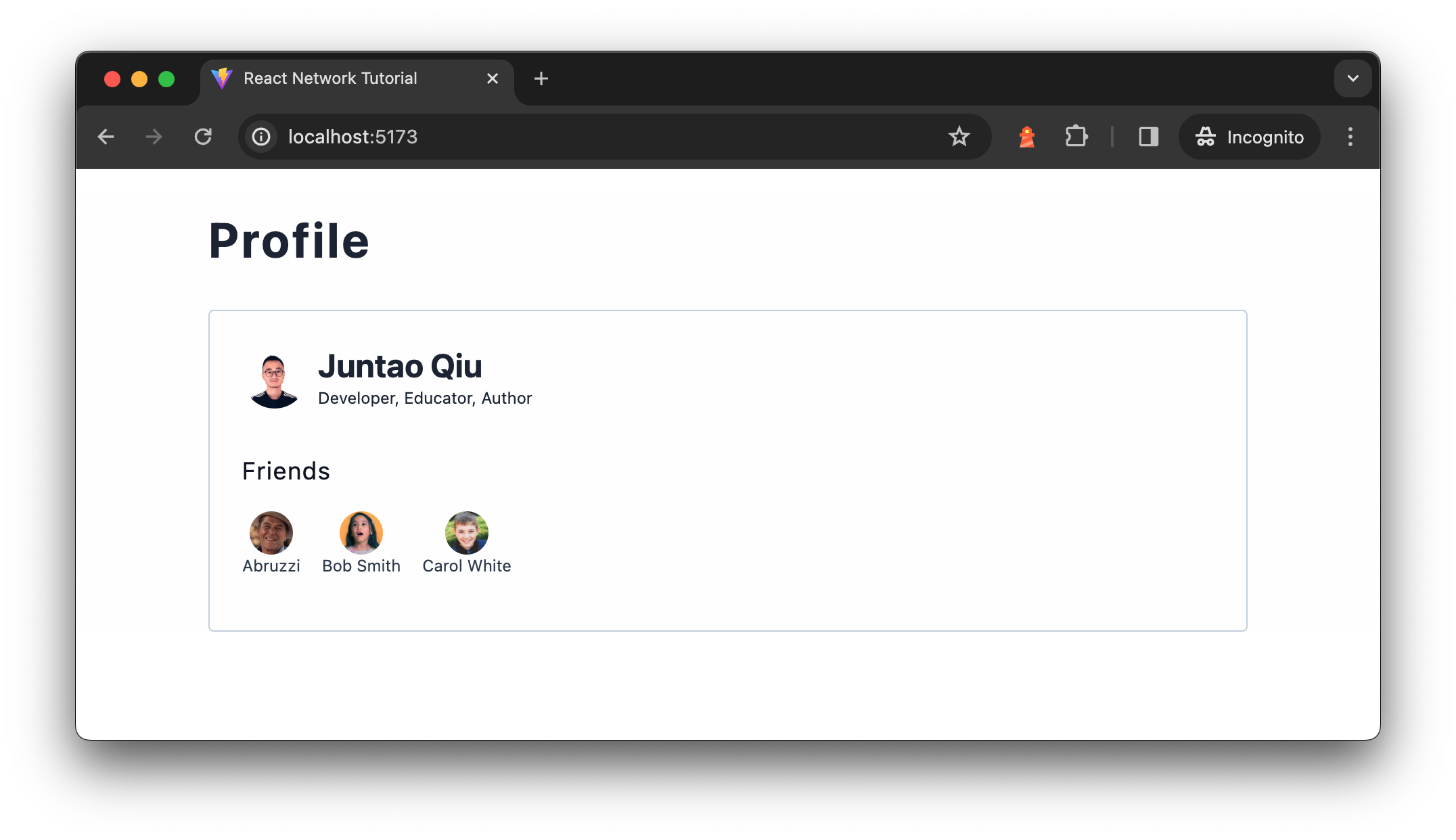
Determine 1: Profile display
The information are from two separate API calls, the consumer transient API
/customers/<id>
returns consumer transient for a given consumer id, which is an easy
object described as follows:
{ "id": "u1", "identify": "Juntao Qiu", "bio": "Developer, Educator, Writer", "pursuits": [ "Technology", "Outdoors", "Travel" ] }
And the good friend API /customers/<id>/mates
endpoint returns a listing of
mates for a given consumer, every checklist merchandise within the response is identical as
the above consumer information. The explanation now we have two endpoints as an alternative of returning
a mates
part of the consumer API is that there are circumstances the place one
might have too many mates (say 1,000), however most individuals do not have many.
This in-balance information construction may be fairly tough, particularly once we
must paginate. The purpose right here is that there are circumstances we have to deal
with a number of community requests.
A short introduction to related React ideas
As this text leverages React for example numerous patterns, I do
not assume a lot about React. Somewhat than anticipating you to spend so much
of time looking for the precise elements within the React documentation, I’ll
briefly introduce these ideas we’ll make the most of all through this
article. When you already perceive what React parts are, and the
use of the
useState
and useEffect
hooks, you could
use this hyperlink to skip forward to the following
part.
For these searching for a extra thorough tutorial, the new React documentation is a superb
useful resource.
What’s a React Part?
In React, parts are the elemental constructing blocks. To place it
merely, a React part is a perform that returns a chunk of UI,
which may be as easy as a fraction of HTML. Think about the
creation of a part that renders a navigation bar:
import React from 'react'; perform Navigation() { return ( <nav> <ol> <li>Dwelling</li> <li>Blogs</li> <li>Books</li> </ol> </nav> ); }
At first look, the combination of JavaScript with HTML tags may appear
unusual (it is known as JSX, a syntax extension to JavaScript. For these
utilizing TypeScript, an analogous syntax known as TSX is used). To make this
code practical, a compiler is required to translate the JSX into legitimate
JavaScript code. After being compiled by Babel,
the code would roughly translate to the next:
perform Navigation() { return React.createElement( "nav", null, React.createElement( "ol", null, React.createElement("li", null, "Dwelling"), React.createElement("li", null, "Blogs"), React.createElement("li", null, "Books") ) ); }
Notice right here the translated code has a perform known as
React.createElement
, which is a foundational perform in
React for creating components. JSX written in React parts is compiled
all the way down to React.createElement
calls behind the scenes.
The essential syntax of React.createElement
is:
React.createElement(sort, [props], [...children])
sort
: A string (e.g., ‘div’, ‘span’) indicating the kind of
DOM node to create, or a React part (class or practical) for
extra subtle constructions.props
: An object containing properties handed to the
factor or part, together with occasion handlers, types, and attributes
likeclassName
andid
.kids
: These elective arguments may be extra
React.createElement
calls, strings, numbers, or any combine
thereof, representing the factor’s kids.
As an example, a easy factor may be created with
React.createElement
as follows:
React.createElement('div', { className: 'greeting' }, 'Hey, world!');
That is analogous to the JSX model:
<div className="greeting">Hey, world!</div>
Beneath the floor, React invokes the native DOM API (e.g.,
doc.createElement("ol")
) to generate DOM components as obligatory.
You may then assemble your customized parts right into a tree, much like
HTML code:
import React from 'react'; import Navigation from './Navigation.tsx'; import Content material from './Content material.tsx'; import Sidebar from './Sidebar.tsx'; import ProductList from './ProductList.tsx'; perform App() { return <Web page />; } perform Web page() { return <Container> <Navigation /> <Content material> <Sidebar /> <ProductList /> </Content material> <Footer /> </Container>; }
In the end, your utility requires a root node to mount to, at
which level React assumes management and manages subsequent renders and
re-renders:
import ReactDOM from "react-dom/shopper"; import App from "./App.tsx"; const root = ReactDOM.createRoot(doc.getElementById('root')); root.render(<App />);
Producing Dynamic Content material with JSX
The preliminary instance demonstrates an easy use case, however
let’s discover how we will create content material dynamically. As an example, how
can we generate a listing of information dynamically? In React, as illustrated
earlier, a part is essentially a perform, enabling us to cross
parameters to it.
import React from 'react'; perform Navigation({ nav }) { return ( <nav> <ol> {nav.map(merchandise => <li key={merchandise}>{merchandise}</li>)} </ol> </nav> ); }
On this modified Navigation
part, we anticipate the
parameter to be an array of strings. We make the most of the map
perform to iterate over every merchandise, reworking them into
<li>
components. The curly braces {}
signify
that the enclosed JavaScript expression must be evaluated and
rendered. For these curious concerning the compiled model of this dynamic
content material dealing with:
perform Navigation(props) { var nav = props.nav; return React.createElement( "nav", null, React.createElement( "ol", null, nav.map(perform(merchandise) { return React.createElement("li", { key: merchandise }, merchandise); }) ) ); }
As an alternative of invoking Navigation
as a daily perform,
using JSX syntax renders the part invocation extra akin to
writing markup, enhancing readability:
// As an alternative of this Navigation(["Home", "Blogs", "Books"]) // We do that <Navigation nav={["Home", "Blogs", "Books"]} />
Parts in React can obtain various information, referred to as props, to
modify their habits, very similar to passing arguments right into a perform (the
distinction lies in utilizing JSX syntax, making the code extra acquainted and
readable to these with HTML information, which aligns effectively with the ability
set of most frontend builders).
import React from 'react'; import Checkbox from './Checkbox'; import BookList from './BookList'; perform App() { let showNewOnly = false; // This flag's worth is often set based mostly on particular logic. const filteredBooks = showNewOnly ? booksData.filter(guide => guide.isNewPublished) : booksData; return ( <div> <Checkbox checked={showNewOnly}> Present New Revealed Books Solely </Checkbox> <BookList books={filteredBooks} /> </div> ); }
On this illustrative code snippet (non-functional however meant to
display the idea), we manipulate the BookList
part’s displayed content material by passing it an array of books. Relying
on the showNewOnly
flag, this array is both all obtainable
books or solely these which might be newly revealed, showcasing how props can
be used to dynamically regulate part output.
Managing Inner State Between Renders: useState
Constructing consumer interfaces (UI) typically transcends the era of
static HTML. Parts regularly must “keep in mind” sure states and
reply to consumer interactions dynamically. As an example, when a consumer
clicks an “Add” button in a Product part, it is necessary to replace
the ShoppingCart part to replicate each the entire worth and the
up to date merchandise checklist.
Within the earlier code snippet, trying to set the
showNewOnly
variable to true
inside an occasion
handler doesn’t obtain the specified impact:
perform App () { let showNewOnly = false; const handleCheckboxChange = () => { showNewOnly = true; // this does not work }; const filteredBooks = showNewOnly ? booksData.filter(guide => guide.isNewPublished) : booksData; return ( <div> <Checkbox checked={showNewOnly} onChange={handleCheckboxChange}> Present New Revealed Books Solely </Checkbox> <BookList books={filteredBooks}/> </div> ); };
This strategy falls quick as a result of native variables inside a perform
part don’t persist between renders. When React re-renders this
part, it does so from scratch, disregarding any adjustments made to
native variables since these don’t set off re-renders. React stays
unaware of the necessity to replace the part to replicate new information.
This limitation underscores the need for React’s
state
. Particularly, practical parts leverage the
useState
hook to recollect states throughout renders. Revisiting
the App
instance, we will successfully keep in mind the
showNewOnly
state as follows:
import React, { useState } from 'react'; import Checkbox from './Checkbox'; import BookList from './BookList'; perform App () { const [showNewOnly, setShowNewOnly] = useState(false); const handleCheckboxChange = () => { setShowNewOnly(!showNewOnly); }; const filteredBooks = showNewOnly ? booksData.filter(guide => guide.isNewPublished) : booksData; return ( <div> <Checkbox checked={showNewOnly} onChange={handleCheckboxChange}> Present New Revealed Books Solely </Checkbox> <BookList books={filteredBooks}/> </div> ); };
The useState
hook is a cornerstone of React’s Hooks system,
launched to allow practical parts to handle inner state. It
introduces state to practical parts, encapsulated by the next
syntax:
const [state, setState] = useState(initialState);
initialState
: This argument is the preliminary
worth of the state variable. It may be a easy worth like a quantity,
string, boolean, or a extra complicated object or array. The
initialState
is simply used in the course of the first render to
initialize the state.- Return Worth:
useState
returns an array with
two components. The primary factor is the present state worth, and the
second factor is a perform that permits updating this worth. Through the use of
array destructuring, we assign names to those returned gadgets,
sometimesstate
andsetState
, although you’ll be able to
select any legitimate variable names. state
: Represents the present worth of the
state. It is the worth that might be used within the part’s UI and
logic.setState
: A perform to replace the state. This perform
accepts a brand new state worth or a perform that produces a brand new state based mostly
on the earlier state. When known as, it schedules an replace to the
part’s state and triggers a re-render to replicate the adjustments.
React treats state as a snapshot; updating it would not alter the
present state variable however as an alternative triggers a re-render. Throughout this
re-render, React acknowledges the up to date state, making certain the
BookList
part receives the right information, thereby
reflecting the up to date guide checklist to the consumer. This snapshot-like
habits of state facilitates the dynamic and responsive nature of React
parts, enabling them to react intuitively to consumer interactions and
different adjustments.
Managing Aspect Results: useEffect
Earlier than diving deeper into our dialogue, it is essential to deal with the
idea of unwanted effects. Negative effects are operations that work together with
the surface world from the React ecosystem. Frequent examples embrace
fetching information from a distant server or dynamically manipulating the DOM,
equivalent to altering the web page title.
React is primarily involved with rendering information to the DOM and does
not inherently deal with information fetching or direct DOM manipulation. To
facilitate these unwanted effects, React offers the useEffect
hook. This hook permits the execution of unwanted effects after React has
accomplished its rendering course of. If these unwanted effects end in information
adjustments, React schedules a re-render to replicate these updates.
The useEffect
Hook accepts two arguments:
- A perform containing the aspect impact logic.
- An elective dependency array specifying when the aspect impact must be
re-invoked.
Omitting the second argument causes the aspect impact to run after
each render. Offering an empty array []
signifies that your impact
doesn’t rely upon any values from props or state, thus not needing to
re-run. Together with particular values within the array means the aspect impact
solely re-executes if these values change.
When coping with asynchronous information fetching, the workflow inside
useEffect
entails initiating a community request. As soon as the information is
retrieved, it’s captured through the useState
hook, updating the
part’s inner state and preserving the fetched information throughout
renders. React, recognizing the state replace, undertakes one other render
cycle to include the brand new information.
Here is a sensible instance about information fetching and state
administration:
import { useEffect, useState } from "react"; sort Consumer = { id: string; identify: string; }; const UserSection = ({ id }) => { const [user, setUser] = useState<Consumer | undefined>(); useEffect(() => { const fetchUser = async () => { const response = await fetch(`/api/customers/${id}`); const jsonData = await response.json(); setUser(jsonData); }; fetchUser(); }, tag:martinfowler.com,2024-05-15:Parallel-Knowledge-Fetching); return <div> <h2>{consumer?.identify}</h2> </div>; };
Within the code snippet above, inside useEffect
, an
asynchronous perform fetchUser
is outlined after which
instantly invoked. This sample is critical as a result of
useEffect
doesn’t immediately assist async capabilities as its
callback. The async perform is outlined to make use of await
for
the fetch operation, making certain that the code execution waits for the
response after which processes the JSON information. As soon as the information is out there,
it updates the part’s state through setUser
.
The dependency array tag:martinfowler.com,2024-05-15:Parallel-Knowledge-Fetching
on the finish of the
useEffect
name ensures that the impact runs once more provided that
id
adjustments, which prevents pointless community requests on
each render and fetches new consumer information when the id
prop
updates.
This strategy to dealing with asynchronous information fetching inside
useEffect
is a typical apply in React improvement, providing a
structured and environment friendly technique to combine async operations into the
React part lifecycle.
As well as, in sensible functions, managing completely different states
equivalent to loading, error, and information presentation is important too (we’ll
see it the way it works within the following part). For instance, take into account
implementing standing indicators inside a Consumer part to replicate
loading, error, or information states, enhancing the consumer expertise by
offering suggestions throughout information fetching operations.
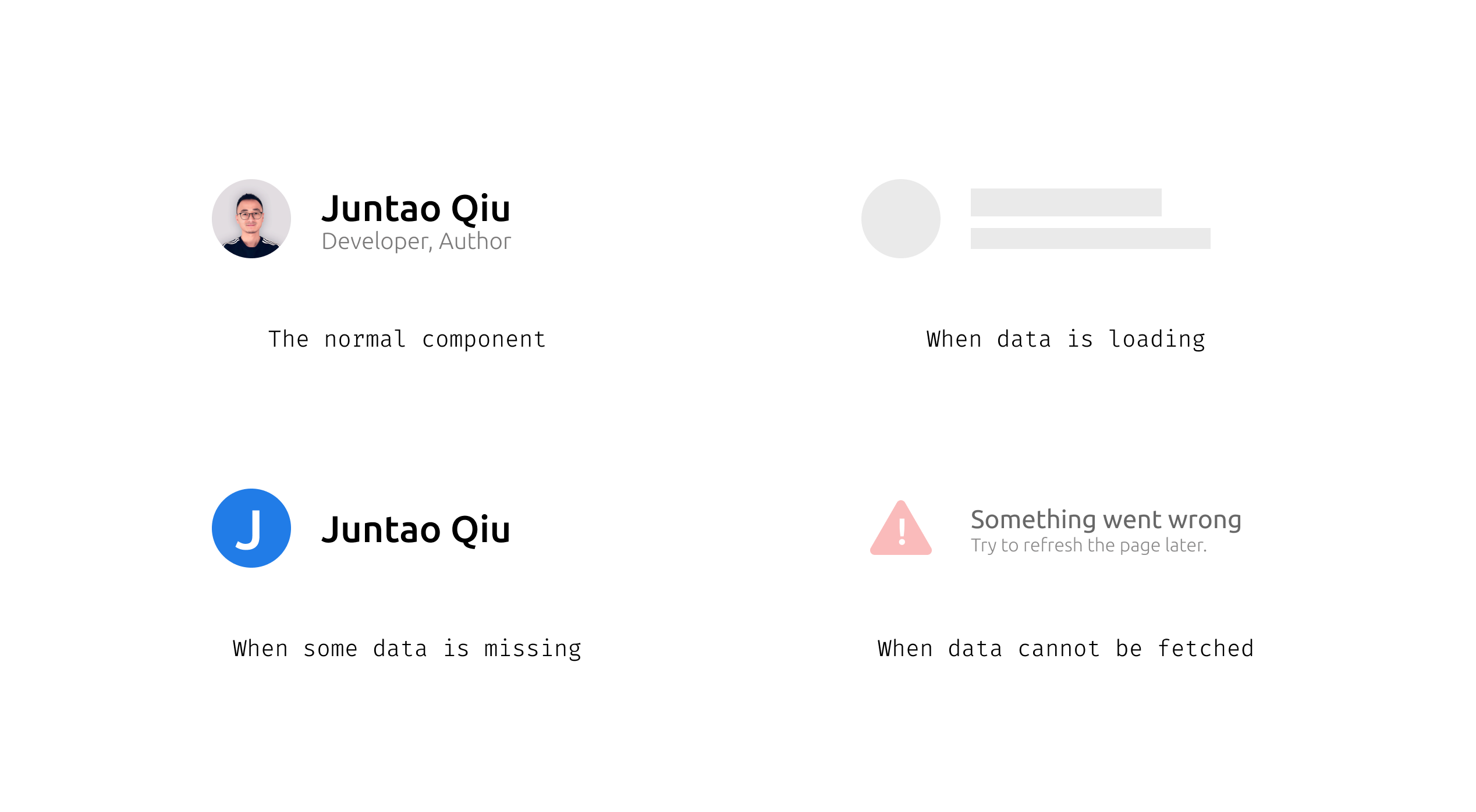
Determine 2: Completely different statuses of a
part
This overview gives only a fast glimpse into the ideas utilized
all through this text. For a deeper dive into extra ideas and
patterns, I like to recommend exploring the new React
documentation or consulting different on-line assets.
With this basis, it is best to now be geared up to hitch me as we delve
into the information fetching patterns mentioned herein.
Implement the Profile part
Let’s create the Profile
part to make a request and
render the outcome. In typical React functions, this information fetching is
dealt with inside a useEffect
block. Here is an instance of how
this is likely to be applied:
import { useEffect, useState } from "react"; const Profile = ({ id }: { id: string }) => { const [user, setUser] = useState<Consumer | undefined>(); useEffect(() => { const fetchUser = async () => { const response = await fetch(`/api/customers/${id}`); const jsonData = await response.json(); setUser(jsonData); }; fetchUser(); }, tag:martinfowler.com,2024-05-15:Parallel-Knowledge-Fetching); return ( <UserBrief consumer={consumer} /> ); };
This preliminary strategy assumes community requests full
instantaneously, which is usually not the case. Actual-world eventualities require
dealing with various community circumstances, together with delays and failures. To
handle these successfully, we incorporate loading and error states into our
part. This addition permits us to offer suggestions to the consumer throughout
information fetching, equivalent to displaying a loading indicator or a skeleton display
if the information is delayed, and dealing with errors after they happen.
Right here’s how the improved part appears to be like with added loading and error
administration:
import { useEffect, useState } from "react"; import { get } from "../utils.ts"; import sort { Consumer } from "../sorts.ts"; const Profile = ({ id }: { id: string }) => { const [loading, setLoading] = useState<boolean>(false); const [error, setError] = useState<Error | undefined>(); const [user, setUser] = useState<Consumer | undefined>(); useEffect(() => { const fetchUser = async () => { strive { setLoading(true); const information = await get<Consumer>(`/customers/${id}`); setUser(information); } catch (e) { setError(e as Error); } lastly { setLoading(false); } }; fetchUser(); }, tag:martinfowler.com,2024-05-15:Parallel-Knowledge-Fetching); if (loading || !consumer) { return <div>Loading...</div>; } return ( <> {consumer && <UserBrief consumer={consumer} />} </> ); };
Now in Profile
part, we provoke states for loading,
errors, and consumer information with useState
. Utilizing
useEffect
, we fetch consumer information based mostly on id
,
toggling loading standing and dealing with errors accordingly. Upon profitable
information retrieval, we replace the consumer state, else show a loading
indicator.
The get
perform, as demonstrated beneath, simplifies
fetching information from a selected endpoint by appending the endpoint to a
predefined base URL. It checks the response’s success standing and both
returns the parsed JSON information or throws an error for unsuccessful requests,
streamlining error dealing with and information retrieval in our utility. Notice
it is pure TypeScript code and can be utilized in different non-React elements of the
utility.
const baseurl = "https://icodeit.com.au/api/v2"; async perform get<T>(url: string): Promise<T> { const response = await fetch(`${baseurl}${url}`); if (!response.okay) { throw new Error("Community response was not okay"); } return await response.json() as Promise<T>; }
React will attempt to render the part initially, however as the information
consumer
isn’t obtainable, it returns “loading…” in a
div
. Then the useEffect
is invoked, and the
request is kicked off. As soon as in some unspecified time in the future, the response returns, React
re-renders the Profile
part with consumer
fulfilled, so now you can see the consumer part with identify, avatar, and
title.
If we visualize the timeline of the above code, you will notice
the next sequence. The browser firstly downloads the HTML web page, and
then when it encounters script tags and elegance tags, it’d cease and
obtain these recordsdata, after which parse them to kind the ultimate web page. Notice
that this can be a comparatively difficult course of, and I’m oversimplifying
right here, however the primary concept of the sequence is right.
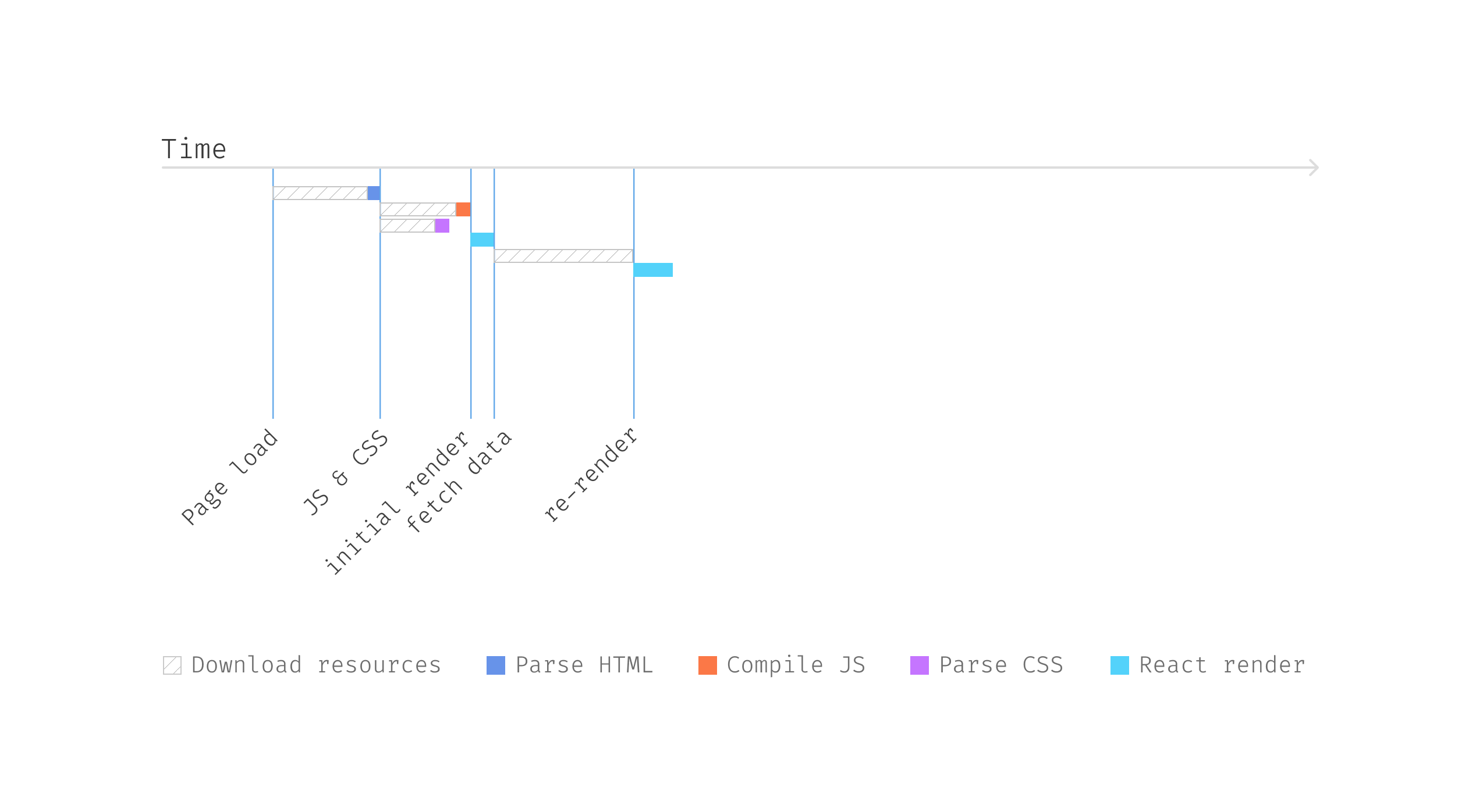
Determine 3: Fetching consumer
information
So React can begin to render solely when the JS are parsed and executed,
after which it finds the useEffect
for information fetching; it has to attend till
the information is out there for a re-render.
Now within the browser, we will see a “loading…” when the applying
begins, after which after just a few seconds (we will simulate such case by add
some delay within the API endpoints) the consumer transient part exhibits up when information
is loaded.
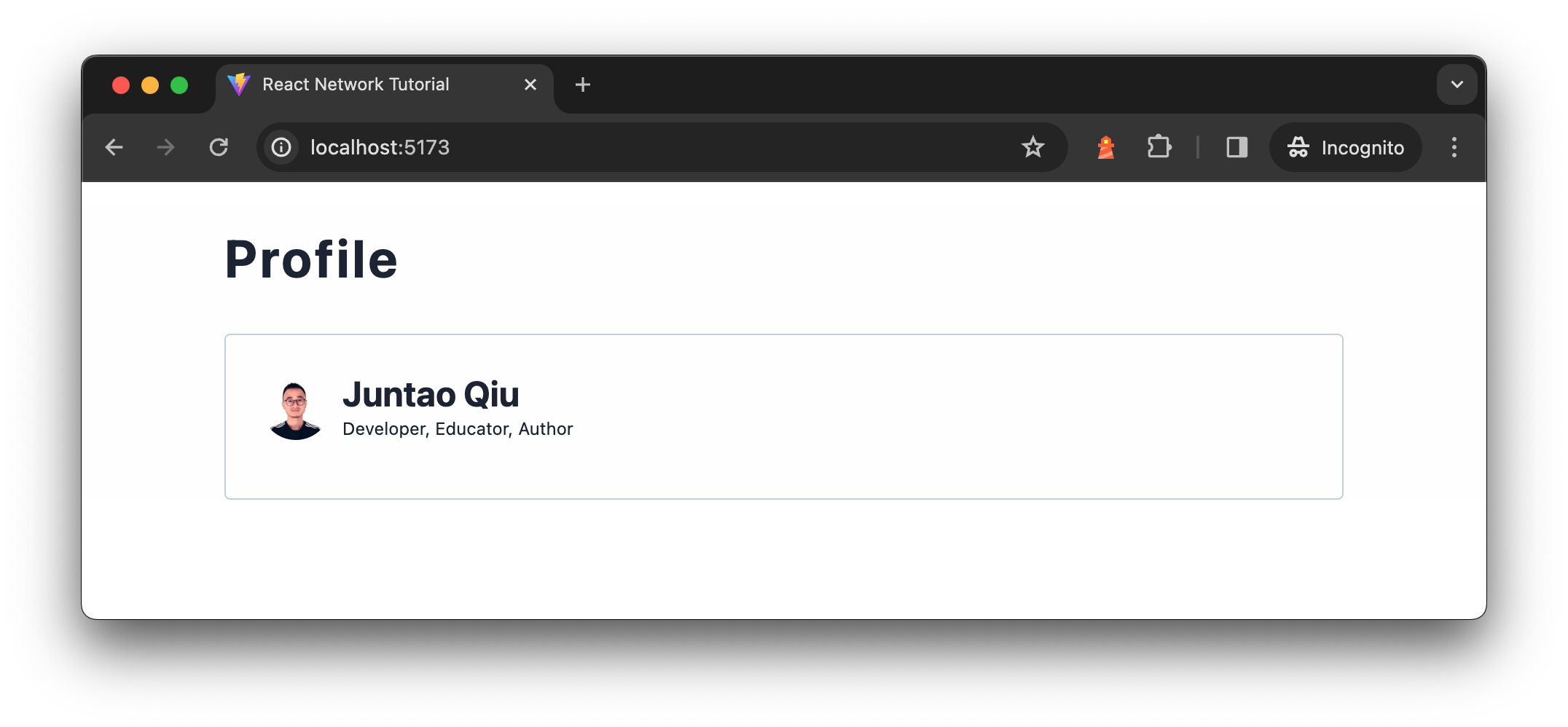
Determine 4: Consumer transient part
This code construction (in useEffect to set off request, and replace states
like loading
and error
correspondingly) is
broadly used throughout React codebases. In functions of normal measurement, it is
widespread to search out quite a few situations of such identical data-fetching logic
dispersed all through numerous parts.
Asynchronous State Handler
Wrap asynchronous queries with meta-queries for the state of the
question.
Distant calls may be gradual, and it is important to not let the UI freeze
whereas these calls are being made. Subsequently, we deal with them asynchronously
and use indicators to indicate {that a} course of is underway, which makes the
consumer expertise higher – realizing that one thing is going on.
Moreover, distant calls may fail on account of connection points,
requiring clear communication of those failures to the consumer. Subsequently,
it is best to encapsulate every distant name inside a handler module that
manages outcomes, progress updates, and errors. This module permits the UI
to entry metadata concerning the standing of the decision, enabling it to show
different info or choices if the anticipated outcomes fail to
materialize.
A easy implementation could possibly be a perform getAsyncStates
that
returns these metadata, it takes a URL as its parameter and returns an
object containing info important for managing asynchronous
operations. This setup permits us to appropriately reply to completely different
states of a community request, whether or not it is in progress, efficiently
resolved, or has encountered an error.
const { loading, error, information } = getAsyncStates(url); if (loading) { // Show a loading spinner } if (error) { // Show an error message } // Proceed to render utilizing the information
The idea right here is that getAsyncStates
initiates the
community request mechanically upon being known as. Nonetheless, this may not
all the time align with the caller’s wants. To supply extra management, we will additionally
expose a fetch
perform throughout the returned object, permitting
the initiation of the request at a extra acceptable time, in accordance with the
caller’s discretion. Moreover, a refetch
perform might
be offered to allow the caller to re-initiate the request as wanted,
equivalent to after an error or when up to date information is required. The
fetch
and refetch
capabilities may be an identical in
implementation, or refetch
may embrace logic to verify for
cached outcomes and solely re-fetch information if obligatory.
const { loading, error, information, fetch, refetch } = getAsyncStates(url); const onInit = () => { fetch(); }; const onRefreshClicked = () => { refetch(); }; if (loading) { // Show a loading spinner } if (error) { // Show an error message } // Proceed to render utilizing the information
This sample offers a flexible strategy to dealing with asynchronous
requests, giving builders the pliability to set off information fetching
explicitly and handle the UI’s response to loading, error, and success
states successfully. By decoupling the fetching logic from its initiation,
functions can adapt extra dynamically to consumer interactions and different
runtime circumstances, enhancing the consumer expertise and utility
reliability.
Implementing Asynchronous State Handler in React with hooks
The sample may be applied in several frontend libraries. For
occasion, we might distill this strategy right into a customized Hook in a React
utility for the Profile part:
import { useEffect, useState } from "react"; import { get } from "../utils.ts"; const useUser = (id: string) => { const [loading, setLoading] = useState<boolean>(false); const [error, setError] = useState<Error | undefined>(); const [user, setUser] = useState<Consumer | undefined>(); useEffect(() => { const fetchUser = async () => { strive { setLoading(true); const information = await get<Consumer>(`/customers/${id}`); setUser(information); } catch (e) { setError(e as Error); } lastly { setLoading(false); } }; fetchUser(); }, tag:martinfowler.com,2024-05-15:Parallel-Knowledge-Fetching); return { loading, error, consumer, }; };
Please word that within the customized Hook, we haven’t any JSX code –
that means it’s very UI free however sharable stateful logic. And the
useUser
launch information mechanically when known as. Throughout the Profile
part, leveraging the useUser
Hook simplifies its logic:
import { useUser } from './useUser.ts'; import UserBrief from './UserBrief.tsx'; const Profile = ({ id }: { id: string }) => { const { loading, error, consumer } = useUser(id); if (loading || !consumer) { return <div>Loading...</div>; } if (error) { return <div>One thing went incorrect...</div>; } return ( <> {consumer && <UserBrief consumer={consumer} />} </> ); };
Generalizing Parameter Utilization
In most functions, fetching various kinds of information—from consumer
particulars on a homepage to product lists in search outcomes and
suggestions beneath them—is a standard requirement. Writing separate
fetch capabilities for every sort of information may be tedious and troublesome to
preserve. A greater strategy is to summary this performance right into a
generic, reusable hook that may deal with numerous information sorts
effectively.
Think about treating distant API endpoints as providers, and use a generic
useService
hook that accepts a URL as a parameter whereas managing all
the metadata related to an asynchronous request:
import { get } from "../utils.ts"; perform useService<T>(url: string) { const [loading, setLoading] = useState<boolean>(false); const [error, setError] = useState<Error | undefined>(); const [data, setData] = useState<T | undefined>(); const fetch = async () => { strive { setLoading(true); const information = await get<T>(url); setData(information); } catch (e) { setError(e as Error); } lastly { setLoading(false); } }; return { loading, error, information, fetch, }; }
This hook abstracts the information fetching course of, making it simpler to
combine into any part that should retrieve information from a distant
supply. It additionally centralizes widespread error dealing with eventualities, equivalent to
treating particular errors in a different way:
import { useService } from './useService.ts'; const { loading, error, information: consumer, fetch: fetchUser, } = useService(`/customers/${id}`);
Through the use of useService, we will simplify how parts fetch and deal with
information, making the codebase cleaner and extra maintainable.
Variation of the sample
A variation of the useUser
can be expose the
fetchUsers
perform, and it doesn’t set off the information
fetching itself:
import { useState } from "react"; const useUser = (id: string) => { // outline the states const fetchUser = async () => { strive { setLoading(true); const information = await get<Consumer>(`/customers/${id}`); setUser(information); } catch (e) { setError(e as Error); } lastly { setLoading(false); } }; return { loading, error, consumer, fetchUser, }; };
After which on the calling web site, Profile
part use
useEffect
to fetch the information and render completely different
states.
const Profile = ({ id }: { id: string }) => { const { loading, error, consumer, fetchUser } = useUser(id); useEffect(() => { fetchUser(); }, []); // render correspondingly };
The benefit of this division is the flexibility to reuse these stateful
logics throughout completely different parts. As an example, one other part
needing the identical information (a consumer API name with a consumer ID) can merely import
the useUser
Hook and make the most of its states. Completely different UI
parts may select to work together with these states in numerous methods,
maybe utilizing different loading indicators (a smaller spinner that
matches to the calling part) or error messages, but the elemental
logic of fetching information stays constant and shared.
When to make use of it
Separating information fetching logic from UI parts can typically
introduce pointless complexity, notably in smaller functions.
Protecting this logic built-in throughout the part, much like the
css-in-js strategy, simplifies navigation and is simpler for some
builders to handle. In my article, Modularizing
React Purposes with Established UI Patterns, I explored
numerous ranges of complexity in utility constructions. For functions
which might be restricted in scope — with just some pages and a number of other information
fetching operations — it is typically sensible and in addition advisable to
preserve information fetching inside the UI parts.
Nonetheless, as your utility scales and the event workforce grows,
this technique could result in inefficiencies. Deep part bushes can gradual
down your utility (we’ll see examples in addition to learn how to tackle
them within the following sections) and generate redundant boilerplate code.
Introducing an Asynchronous State Handler can mitigate these points by
decoupling information fetching from UI rendering, enhancing each efficiency
and maintainability.
It’s essential to stability simplicity with structured approaches as your
undertaking evolves. This ensures your improvement practices stay
efficient and attentive to the applying’s wants, sustaining optimum
efficiency and developer effectivity whatever the undertaking
scale.
Implement the Buddies checklist
Now let’s take a look on the second part of the Profile – the good friend
checklist. We will create a separate part Buddies
and fetch information in it
(through the use of a useService customized hook we outlined above), and the logic is
fairly much like what we see above within the Profile
part.
const Buddies = ({ id }: { id: string }) => { const { loading, error, information: mates } = useService(`/customers/${id}/mates`); // loading & error dealing with... return ( <div> <h2>Buddies</h2> <div> {mates.map((consumer) => ( // render consumer checklist ))} </div> </div> ); };
After which within the Profile part, we will use Buddies as a daily
part, and cross in id
as a prop:
const Profile = ({ id }: { id: string }) => { //... return ( <> {consumer && <UserBrief consumer={consumer} />} <Buddies id={id} /> </> ); };
The code works high-quality, and it appears to be like fairly clear and readable,
UserBrief
renders a consumer
object handed in, whereas
Buddies
handle its personal information fetching and rendering logic
altogether. If we visualize the part tree, it will be one thing like
this:
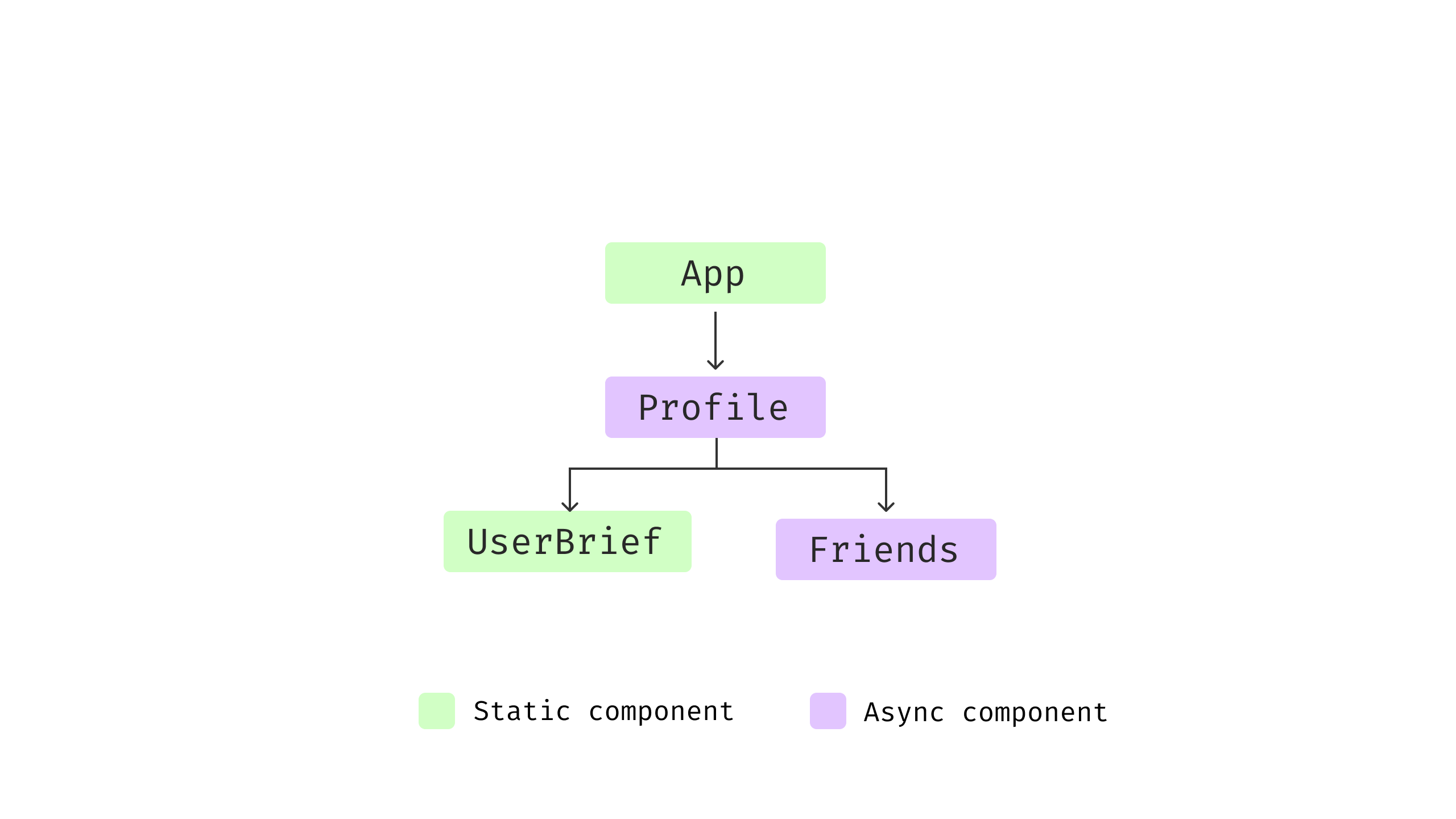
Determine 5: Part construction
Each the Profile
and Buddies
have logic for
information fetching, loading checks, and error dealing with. Since there are two
separate information fetching calls, and if we have a look at the request timeline, we
will discover one thing fascinating.
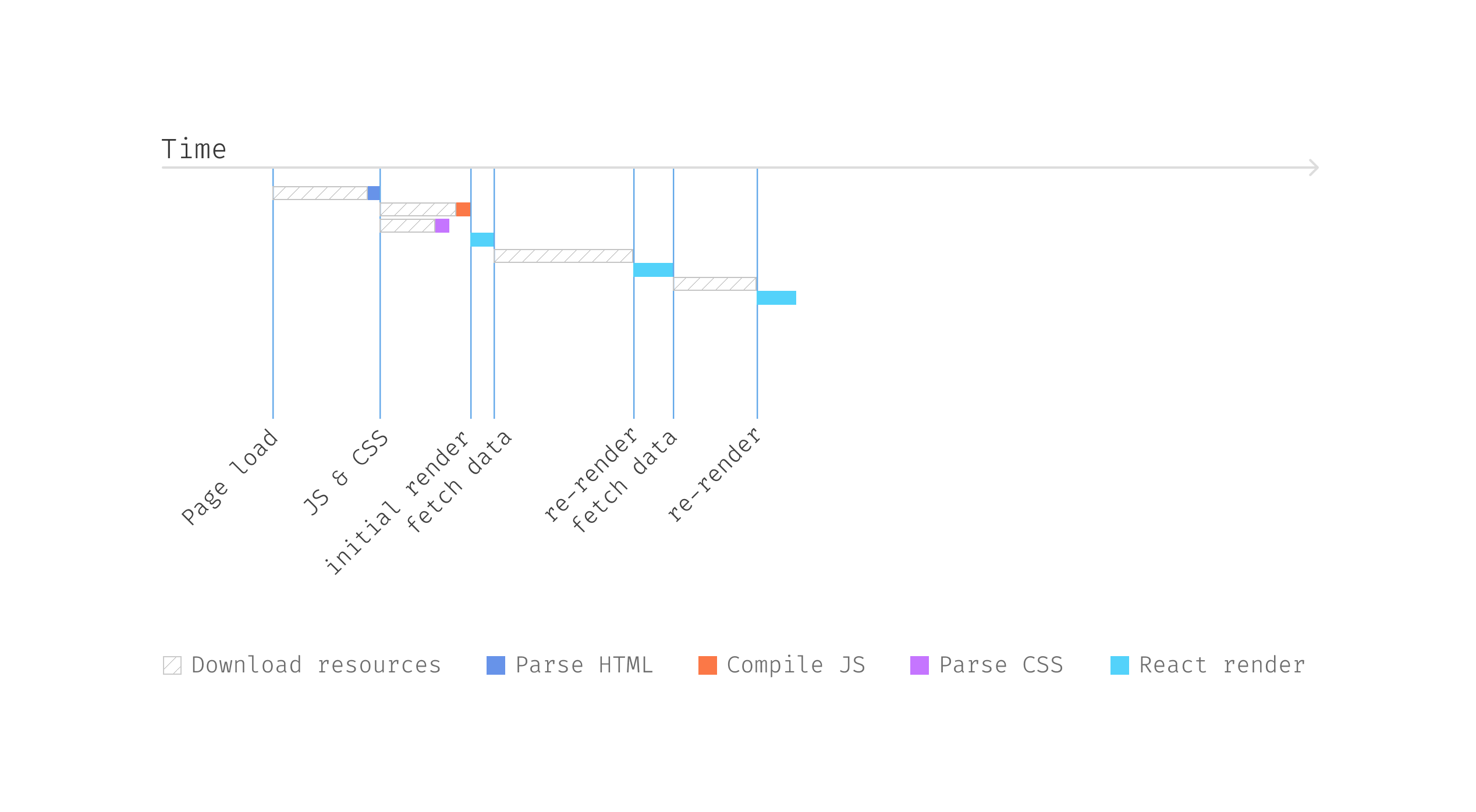
Determine 6: Request waterfall
The Buddies
part will not provoke information fetching till the consumer
state is about. That is known as the Fetch-On-Render strategy,
the place the preliminary rendering is paused as a result of the information is not obtainable,
requiring React to attend for the information to be retrieved from the server
aspect.
This ready interval is considerably inefficient, contemplating that whereas
React’s rendering course of solely takes just a few milliseconds, information fetching can
take considerably longer, typically seconds. Consequently, the Buddies
part spends most of its time idle, ready for information. This state of affairs
results in a standard problem referred to as the Request Waterfall, a frequent
prevalence in frontend functions that contain a number of information fetching
operations.
Parallel Knowledge Fetching
Run distant information fetches in parallel to reduce wait time
Think about once we construct a bigger utility {that a} part that
requires information may be deeply nested within the part tree, to make the
matter worse these parts are developed by completely different groups, it’s exhausting
to see whom we’re blocking.
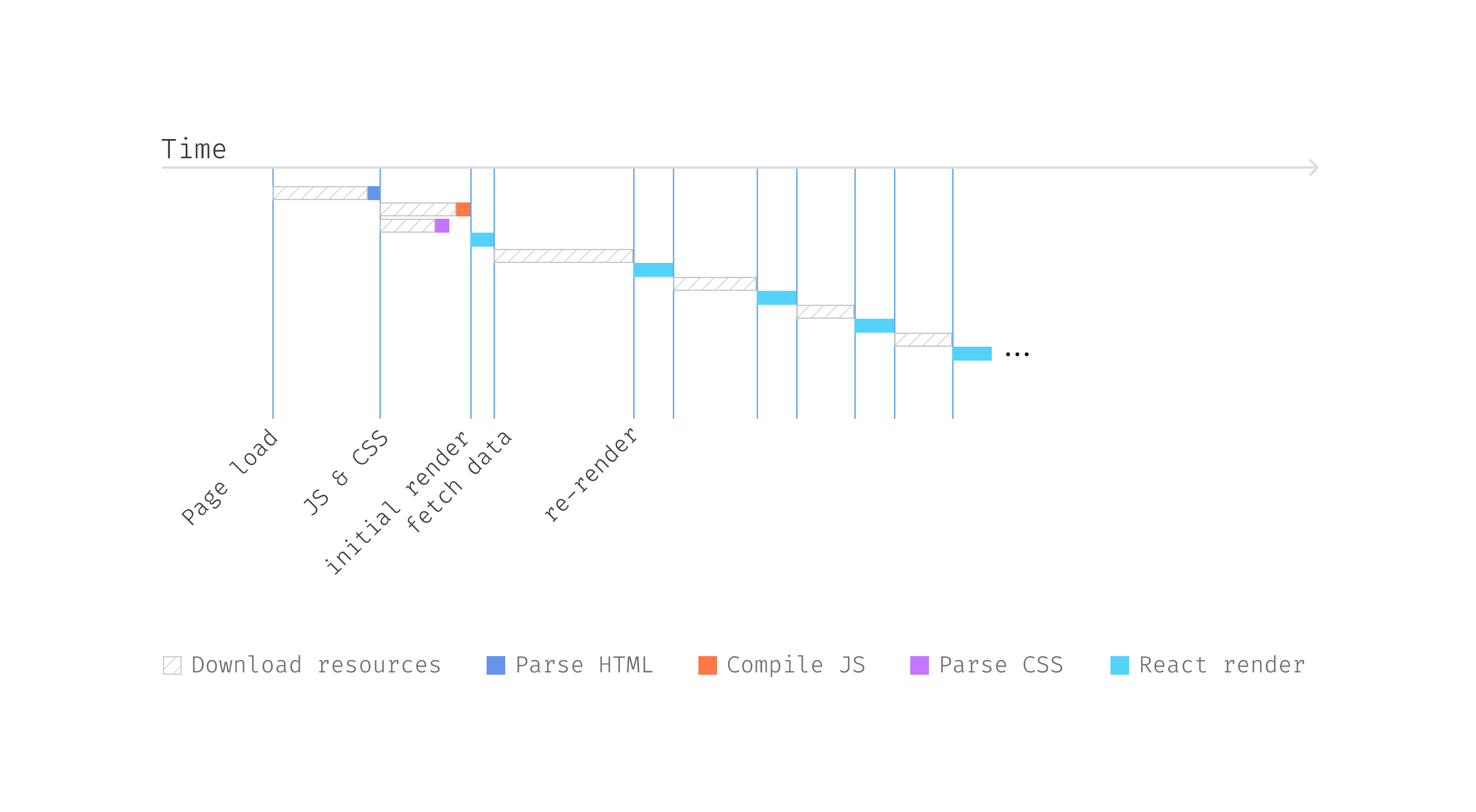
Determine 7: Request waterfall
Request Waterfalls can degrade consumer
expertise, one thing we goal to keep away from. Analyzing the information, we see that the
consumer API and mates API are unbiased and may be fetched in parallel.
Initiating these parallel requests turns into important for utility
efficiency.
One strategy is to centralize information fetching at a better degree, close to the
root. Early within the utility’s lifecycle, we begin all information fetches
concurrently. Parts depending on this information wait just for the
slowest request, sometimes leading to quicker general load instances.
We might use the Promise API Promise.all
to ship
each requests for the consumer’s primary info and their mates checklist.
Promise.all
is a JavaScript technique that permits for the
concurrent execution of a number of guarantees. It takes an array of guarantees
as enter and returns a single Promise that resolves when the entire enter
guarantees have resolved, offering their outcomes as an array. If any of the
guarantees fail, Promise.all
instantly rejects with the
cause of the primary promise that rejects.
As an example, on the utility’s root, we will outline a complete
information mannequin:
sort ProfileState = { consumer: Consumer; mates: Consumer[]; }; const getProfileData = async (id: string) => Promise.all([ get<User>(`/users/${id}`), get<User[]>(`/customers/${id}/mates`), ]); const App = () => { // fetch information on the very begining of the applying launch const onInit = () => { const [user, friends] = await getProfileData(id); } // render the sub tree correspondingly }
Implementing Parallel Knowledge Fetching in React
Upon utility launch, information fetching begins, abstracting the
fetching course of from subcomponents. For instance, in Profile part,
each UserBrief and Buddies are presentational parts that react to
the handed information. This fashion we might develop these part individually
(including types for various states, for instance). These presentational
parts usually are straightforward to check and modify as now we have separate the
information fetching and rendering.
We will outline a customized hook useProfileData
that facilitates
parallel fetching of information associated to a consumer and their mates through the use of
Promise.all
. This technique permits simultaneous requests, optimizing the
loading course of and structuring the information right into a predefined format identified
as ProfileData
.
Right here’s a breakdown of the hook implementation:
import { useCallback, useEffect, useState } from "react"; sort ProfileData = { consumer: Consumer; mates: Consumer[]; }; const useProfileData = (id: string) => { const [loading, setLoading] = useState<boolean>(false); const [error, setError] = useState<Error | undefined>(undefined); const [profileState, setProfileState] = useState<ProfileData>(); const fetchProfileState = useCallback(async () => { strive { setLoading(true); const [user, friends] = await Promise.all([ get<User>(`/users/${id}`), get<User[]>(`/customers/${id}/mates`), ]); setProfileState({ consumer, mates }); } catch (e) { setError(e as Error); } lastly { setLoading(false); } }, tag:martinfowler.com,2024-05-15:Parallel-Knowledge-Fetching); return { loading, error, profileState, fetchProfileState, }; };
This hook offers the Profile
part with the
obligatory information states (loading
, error
,
profileState
) together with a fetchProfileState
perform, enabling the part to provoke the fetch operation as
wanted. Notice right here we use useCallback
hook to wrap the async
perform for information fetching. The useCallback hook in React is used to
memoize capabilities, making certain that the identical perform occasion is
maintained throughout part re-renders until its dependencies change.
Much like the useEffect, it accepts the perform and a dependency
array, the perform will solely be recreated if any of those dependencies
change, thereby avoiding unintended habits in React’s rendering
cycle.
The Profile
part makes use of this hook and controls the information fetching
timing through useEffect
:
const Profile = ({ id }: { id: string }) => { const { loading, error, profileState, fetchProfileState } = useProfileData(id); useEffect(() => { fetchProfileState(); }, [fetchProfileState]); if (loading) { return <div>Loading...</div>; } if (error) { return <div>One thing went incorrect...</div>; } return ( <> {profileState && ( <> <UserBrief consumer={profileState.consumer} /> <Buddies customers={profileState.mates} /> </> )} </> ); };
This strategy is also referred to as Fetch-Then-Render, suggesting that the goal
is to provoke requests as early as doable throughout web page load.
Subsequently, the fetched information is utilized to drive React’s rendering of
the applying, bypassing the necessity to handle information fetching amidst the
rendering course of. This technique simplifies the rendering course of,
making the code simpler to check and modify.
And the part construction, if visualized, can be just like the
following illustration
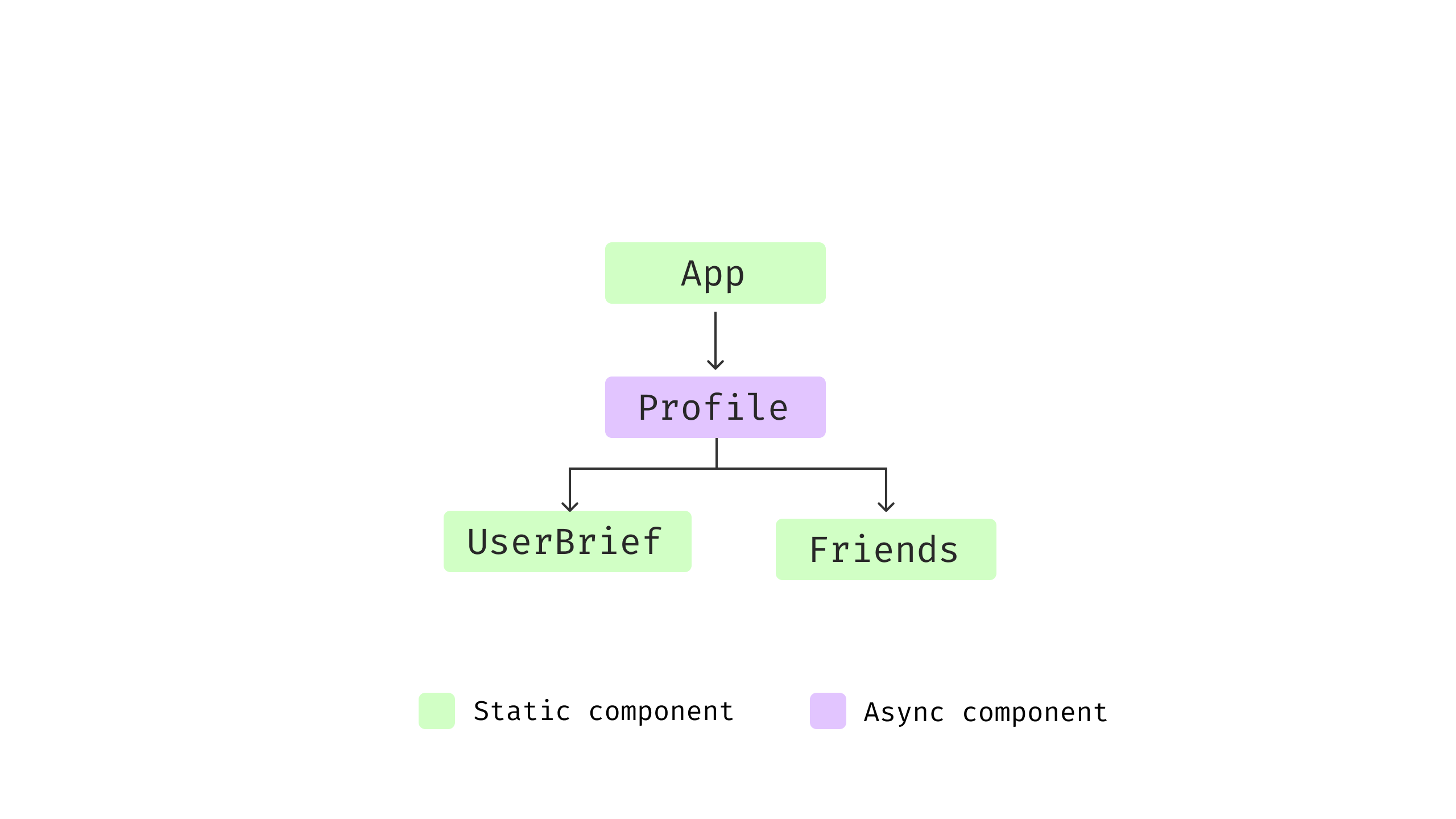
Determine 8: Part construction after refactoring
And the timeline is way shorter than the earlier one as we ship two
requests in parallel. The Buddies
part can render in just a few
milliseconds as when it begins to render, the information is already prepared and
handed in.
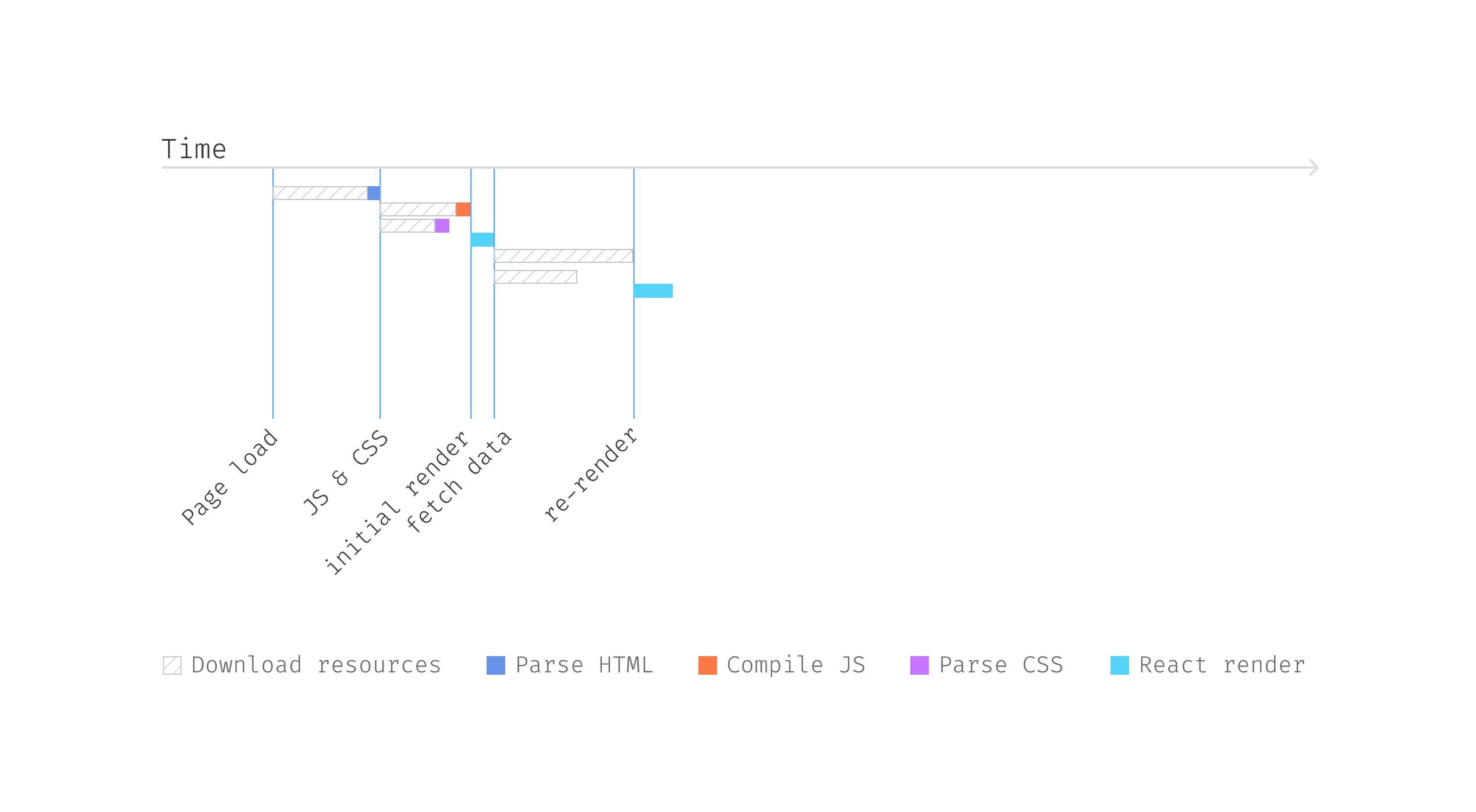
Determine 9: Parallel requests
Notice that the longest wait time relies on the slowest community
request, which is way quicker than the sequential ones. And if we might
ship as many of those unbiased requests on the identical time at an higher
degree of the part tree, a greater consumer expertise may be
anticipated.
As functions develop, managing an rising variety of requests at
root degree turns into difficult. That is notably true for parts
distant from the foundation, the place passing down information turns into cumbersome. One
strategy is to retailer all information globally, accessible through capabilities (like
Redux or the React Context API), avoiding deep prop drilling.
When to make use of it
Operating queries in parallel is helpful at any time when such queries could also be
gradual and do not considerably intervene with every others’ efficiency.
That is normally the case with distant queries. Even when the distant
machine’s I/O and computation is quick, there’s all the time potential latency
points within the distant calls. The primary drawback for parallel queries
is setting them up with some sort of asynchronous mechanism, which can be
troublesome in some language environments.
The primary cause to not use parallel information fetching is once we do not
know what information must be fetched till we have already fetched some
information. Sure eventualities require sequential information fetching on account of
dependencies between requests. As an example, take into account a state of affairs on a
Profile
web page the place producing a customized advice feed
relies on first buying the consumer’s pursuits from a consumer API.
Here is an instance response from the consumer API that features
pursuits:
{ "id": "u1", "identify": "Juntao Qiu", "bio": "Developer, Educator, Writer", "pursuits": [ "Technology", "Outdoors", "Travel" ] }
In such circumstances, the advice feed can solely be fetched after
receiving the consumer’s pursuits from the preliminary API name. This
sequential dependency prevents us from using parallel fetching, as
the second request depends on information obtained from the primary.
Given these constraints, it turns into necessary to debate different
methods in asynchronous information administration. One such technique is
Fallback Markup. This strategy permits builders to specify what
information is required and the way it must be fetched in a manner that clearly
defines dependencies, making it simpler to handle complicated information
relationships in an utility.
One other instance of when arallel Knowledge Fetching is just not relevant is
that in eventualities involving consumer interactions that require real-time
information validation.
Think about the case of a listing the place every merchandise has an “Approve” context
menu. When a consumer clicks on the “Approve” choice for an merchandise, a dropdown
menu seems providing selections to both “Approve” or “Reject.” If this
merchandise’s approval standing could possibly be modified by one other admin concurrently,
then the menu choices should replicate essentially the most present state to keep away from
conflicting actions.
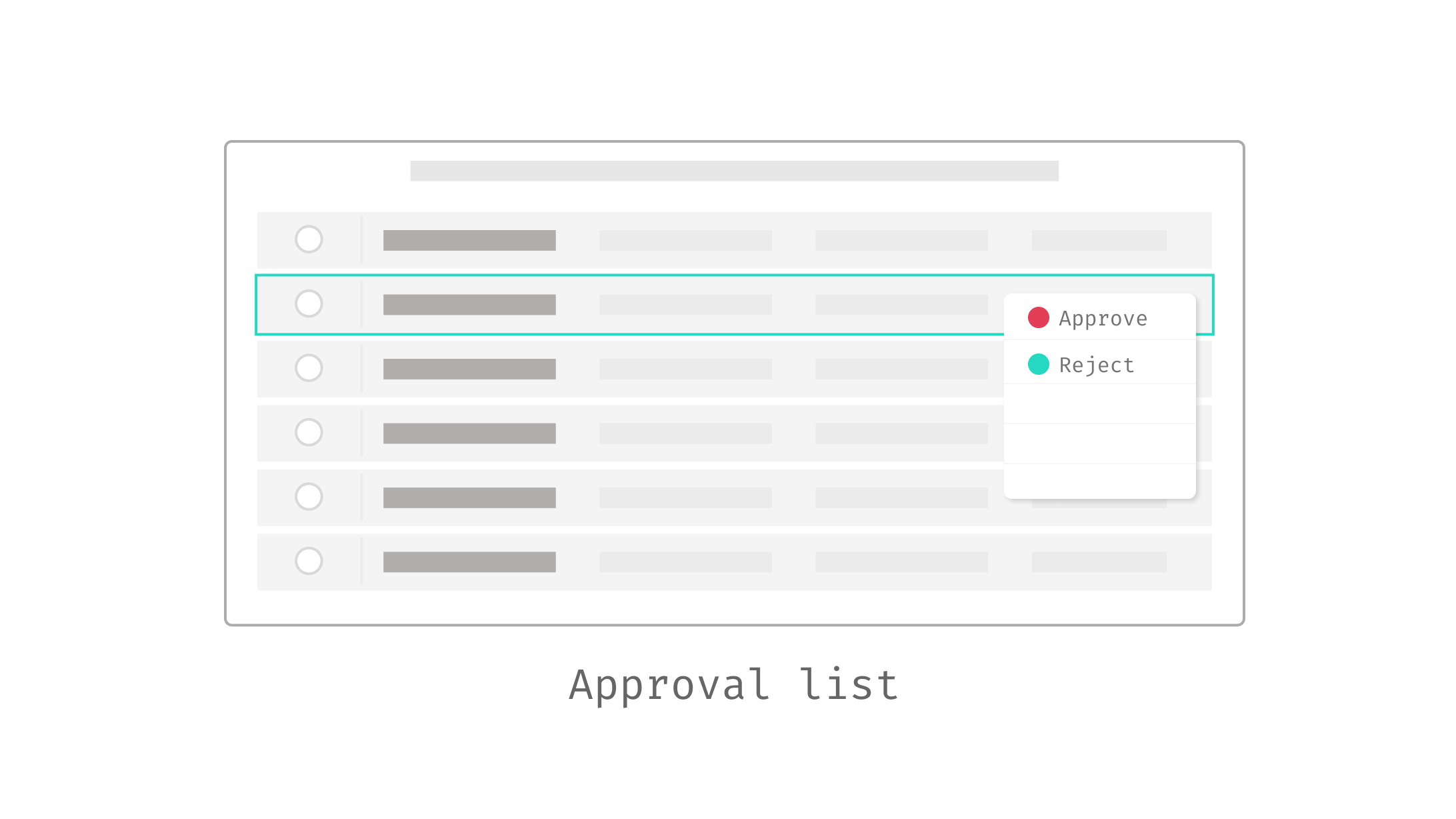
Determine 10: The approval checklist that require in-time
states
To deal with this, a service name is initiated every time the context
menu is activated. This service fetches the newest standing of the merchandise,
making certain that the dropdown is constructed with essentially the most correct and
present choices obtainable at that second. Consequently, these requests
can’t be made in parallel with different data-fetching actions for the reason that
dropdown’s contents rely solely on the real-time standing fetched from
the server.
Noise Diva Smartwatch with Diamond Cut dial, Glossy Metallic Finish, AMOLED Display, Mesh Metal and Leather Strap Options, 100+ Watch Faces, Female Cycle Tracker Smart Watch for Women (Gold Link)
₹2,999.00 (as of May 14, 2024 14:12 GMT +00:00 - More infoProduct prices and availability are accurate as of the date/time indicated and are subject to change. Any price and availability information displayed on [relevant Amazon Site(s), as applicable] at the time of purchase will apply to the purchase of this product.)Samsung Original 25W Single Port, Type-C Fast Charger, (Cable not Included), White
₹1,297.00 (as of May 14, 2024 14:12 GMT +00:00 - More infoProduct prices and availability are accurate as of the date/time indicated and are subject to change. Any price and availability information displayed on [relevant Amazon Site(s), as applicable] at the time of purchase will apply to the purchase of this product.)Ambrane Unbreakable 3A Fast Charging 1.5m Braided Type C Cable for Smartphones, Tablets, Laptops & other Type C devices, 480Mbps Data Sync, Quick Charge 3.0 (RCT15A, Black)
₹129.00 (as of May 14, 2024 14:12 GMT +00:00 - More infoProduct prices and availability are accurate as of the date/time indicated and are subject to change. Any price and availability information displayed on [relevant Amazon Site(s), as applicable] at the time of purchase will apply to the purchase of this product.)Redmi 13C 5G (Startrail Green, 4GB RAM, 128GB Storage) | MediaTek Dimensity 6100+ 5G | 90Hz Display
₹10,499.00 (as of May 14, 2024 14:12 GMT +00:00 - More infoProduct prices and availability are accurate as of the date/time indicated and are subject to change. Any price and availability information displayed on [relevant Amazon Site(s), as applicable] at the time of purchase will apply to the purchase of this product.)realme NARZO 70x 5G (Forest Green, 6GB RAM,128GB Storage| 120Hz Ultra Smooth Display | Dimensity 6100+ 6nm 5G | 50MP AI Camera | 45W Charger in The Box
₹13,499.00 (as of May 14, 2024 14:12 GMT +00:00 - More infoProduct prices and availability are accurate as of the date/time indicated and are subject to change. Any price and availability information displayed on [relevant Amazon Site(s), as applicable] at the time of purchase will apply to the purchase of this product.)Ambrane Unbreakable 3A Fast Charging 1.5m Braided Type C Cable for Smartphones, Tablets, Laptops & other Type C devices, 480Mbps Data Sync, Quick Charge 3.0 (RCT15A, Black)
₹129.00 (as of May 14, 2024 14:12 GMT +00:00 - More infoProduct prices and availability are accurate as of the date/time indicated and are subject to change. Any price and availability information displayed on [relevant Amazon Site(s), as applicable] at the time of purchase will apply to the purchase of this product.)Portronics Konnect L POR-1403 Fast Charging 3A Type-C Cable 1.2 Meter with Charge & Sync Function for All Type-C Devices (White)
₹119.00 (as of May 14, 2024 14:12 GMT +00:00 - More infoProduct prices and availability are accurate as of the date/time indicated and are subject to change. Any price and availability information displayed on [relevant Amazon Site(s), as applicable] at the time of purchase will apply to the purchase of this product.)Ambrane Unbreakable 60W Fast Charging 1.5M Braided Type C to Type C Cable for Smartphones, Tablets, Laptops & other Type C devices, PD Technology, 480Mbps Data Sync (RCTT15, Black)
₹159.00 (as of May 14, 2024 14:12 GMT +00:00 - More infoProduct prices and availability are accurate as of the date/time indicated and are subject to change. Any price and availability information displayed on [relevant Amazon Site(s), as applicable] at the time of purchase will apply to the purchase of this product.)Callas Multipurpose Foldable Laptop Table with Cup Holder | Drawer | Mac Holder | Study Table, Breakfast Table, Foldable and Portable/Ergonomic & Rounded Edges/Non-Slip Legs (WA-27-Black) | Metal
₹497.00 (as of May 14, 2024 14:12 GMT +00:00 - More infoProduct prices and availability are accurate as of the date/time indicated and are subject to change. Any price and availability information displayed on [relevant Amazon Site(s), as applicable] at the time of purchase will apply to the purchase of this product.)Zebronics-NS1500 Laptop Stand Featuring Foldable Design, Anti-Slip Silicone Rubber Pads, Supports Maximum of 5kgs Weight Tabletop
₹299.00 (as of May 14, 2024 14:12 GMT +00:00 - More infoProduct prices and availability are accurate as of the date/time indicated and are subject to change. Any price and availability information displayed on [relevant Amazon Site(s), as applicable] at the time of purchase will apply to the purchase of this product.)Auto Amazon Links: No products found.