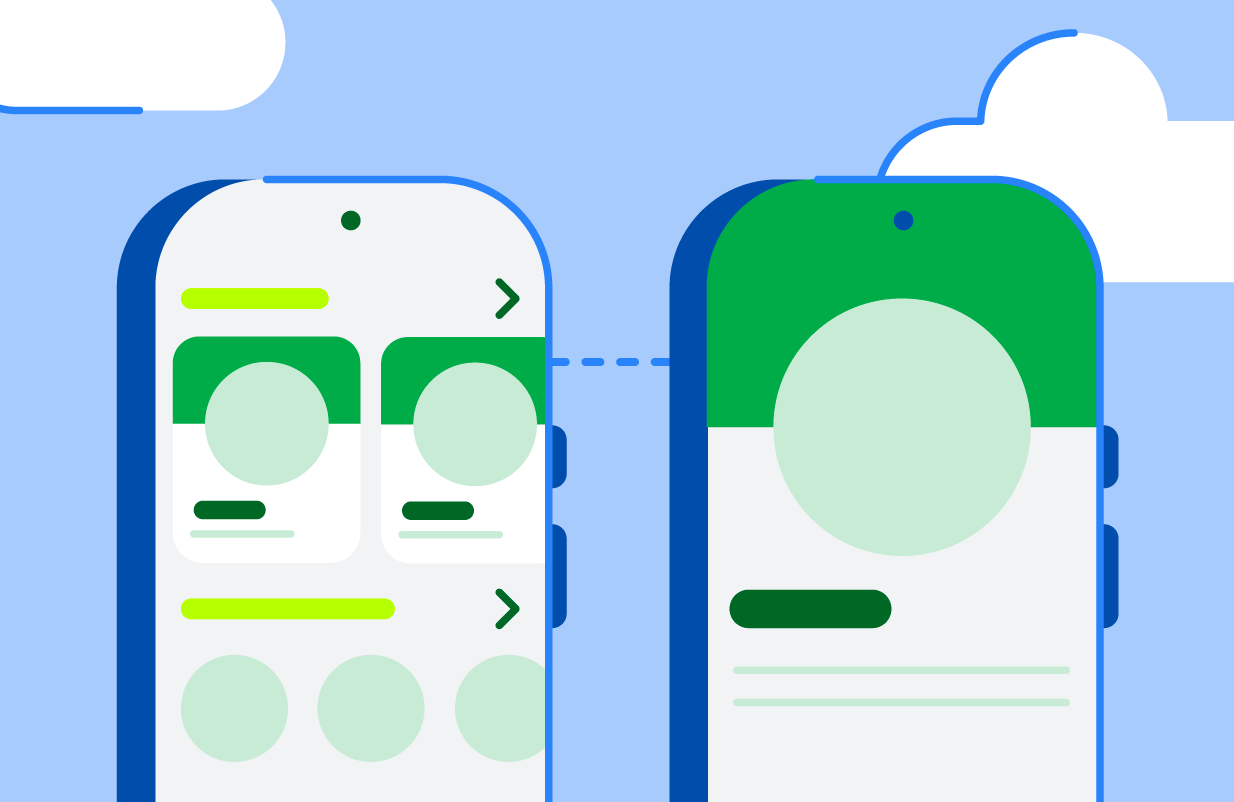
Posted by Ben Trengrove – Developer Relations Engineer, and Nick Butcher – Product Supervisor for Jetpack Compose
At Google I/O 2024, we introduced the upcoming June ‘24 Jetpack Compose launch, filled with options designed to make Android improvement quicker and simpler, it doesn’t matter what kind issue you’re constructing for. We additionally introduced expanded Compose assist throughout the ecosystem of Android gadgets. This publish gives an outline of all of the bulletins, in case you missed any.
Compose June ‘24 launch
Compose is Android’s fashionable, native UI toolkit that’s utilized by apps reminiscent of Threads, Google Drive, and SoundCloud. This launch provides main options you may have been asking for, together with shared component transitions and checklist reordering animations, and improves efficiency.
Shared component transitions
Modifier.sharedElement() and Modifier.sharedBounds() have been added to Compose. They help you create stunning transitions between screens with finer management than the View system allowed. Wrap your AnimatedContent composables in SharedTransitionLayout after which add the Modifier.sharedElement() or Modifier.sharedBounds() onto the content material that you just’d prefer to share between screens. Compose will then discover composables with matching keys and easily transition between them.
Navigation Compose and predictive again animations in Android 15 additionally work easily along with shared component transitions. This lets you create display transitions immediately tied to the navigation gesture, which gives a top quality person expertise.
Take a look at the documentation for extra info.
Lazy checklist merchandise animations
Lazy row and column now have the flexibility to routinely animate the insertion, elimination, and reordering of things. Simply add Modifier.animateItem() to your checklist gadgets and adjustments will routinely animate. You too can customise the animation by offering completely different animation specs.
Take a look at the documentation for the directions.
Textual content
Textual content now helps inline hyperlinks and primary HTML formatting by way of the brand new AnnotatedString.fromHtml() extension. This converts primary HTML formatting, together with hyperlinks to an AnnotatedString, which might then be rendered by Compose.
BasicTextField beneficial properties a brand new overload that accepts a TextFieldState; a brand new wealthy state object that enables extra strong person experiences (reminiscent of wealthy content material like keyboard gifs) and will be hoisted out of the element and up to date asynchronously.
Word: Materials TextField will likely be up to date to make use of the brand new BasicTextField in a subsequent launch, however for those who’d prefer to attempt it out in your apps, see the documentation.
Contextual Movement Layouts
A generally requested structure is a lazy circulation structure that solely composes the content material that may match. When you’ve got ever wanted this performance, ContextualFlowRow and ContextualFlowColumn are for you. Given a max variety of strains, the circulation structure will compose as many gadgets that may match after which present you the context of what was rendered. With this context, you’ll be able to then render a customized increase indicator that exhibits a depend of remaining gadgets.
@OptIn(ExperimentalLayoutApi::class)
@Composable
personal enjoyable SuggestedSnacks(
snacks: Record<Snack>,
onSnackClick: (Snack) -> Unit,
modifier: Modifier = Modifier
) {
var maxLines by bear in mind { mutableIntStateOf(1) }
ContextualFlowRow(
maxLines = maxLines,
overflow = ContextualFlowRowOverflow.expandIndicator {
val remainingItems = totalItemCount - shownItemCount
MyOverflowIndicator(
remainingItems = remainingItems,
modifier = Modifier
.clickable {
// Increase the max strains on click on
maxLines += 1
}
)
},
itemCount = snacks.measurement
) { index ->
val snack = snacks[index]
SnackItem(snack, onSnackClick)
}
}
Efficiency enhancements
Jetpack Compose continues to get quicker with each launch. We’re happy to report that we now have improved the time to first pixel benchmark of our Jetsnack pattern by 17% in comparison with the January launch. We gained these enhancements just by updating the pattern app to the brand new Compose model. The chart under exhibits the advance in Jetsnack with every Compose launch, for the reason that August 2023 launch we now have practically halved the time to first pixel drawn of Jetsnack.
The indication APIs have been rewritten to permit for lazy creation of ripples, which is essential for scroll efficiency. For many apps, there ought to be no adjustments required, however upgrading may introduce breaking adjustments you probably have customized indications. For assist with the migration, see the brand new documentation.
We proceed to enhance the effectivity of the Compose runtime, which accelerates all Compose code in your app. We’ve re-engineered the slot desk to a extra environment friendly knowledge construction, and we’re additionally producing extra environment friendly code by detecting and eradicating unused composition teams. Moreover, there are new APIs that now help you customise the habits of lazy structure prefetching.
Robust skipping mode
Robust skipping mode has graduated from experimental standing and is now production-ready—the upcoming 2.0.20 compiler launch will allow this function by default. Robust skipping mode simplifies composable skipping by permitting composables with unstable parameters to be skipped. This implies extra composables will skip routinely, lowering the necessity to manually annotate lessons as steady. The Now In Android pattern noticed a 20% enchancment in residence display recomposition time when enabling sturdy skipping. For extra particulars see the documentation.
Compose compiler transferring to the Kotlin repository
In case you missed our earlier announcement, the Compose compiler will likely be hosted within the Kotlin repository from Kotlin 2.0. This implies you’ll not have to attend for an identical Compose compiler to be launched to improve your Kotlin model. For full particulars on this transformation and to be taught in regards to the new Compose Compiler Gradle plugin, see our announcement weblog publish.
Secure APIs
We proceed to stabilize experimental APIs and take away experimental annotations. Notable APIs for this launch embody Pager, AnchoredDraggable, SegmentedButton, SwipeToDismissBox, Slider, and extra.
Compose assist throughout Android kind components
We proceed to increase Jetpack Compose to all Android kind components to make it quicker and simpler to construct stunning Android apps, it doesn’t matter what Android kind issue you’re focusing on. At I/O this 12 months we introduced various updates to assist constructing layouts that adapt throughout display sizes, Compose for TV reaching 1.0 Beta and updates to Compose for Put on OS.
Jetpack Libraries
Kind secure navigation in Navigation Compose
As of Jetpack Navigation 2.8.0-alpha08, the Navigation Part has a full sort secure system based mostly on Kotlin Serialization for outlining your navigation graph when utilizing our Kotlin DSL, designed to work finest with integrations like Navigation Compose.
Now, you’ll be able to outline your navigation locations and parameters as serializable objects.
// Outline a house vacation spot that does not take any arguments
@Serializable
object Dwelling
// Outline a profile vacation spot that takes an ID
@Serializable
knowledge class Profile(val id: String)
Then, when defining your NavGraph, passing the article is sufficient. No extra route strings!
NavHost(navController, startDestination = Dwelling) {
composable<Dwelling> {
HomeScreen(onNavigateToProfile = { id ->
navController.navigate(Profile(id))
})
}
composable<Profile> { backStackEntry ->
val profile: Profile = backStackEntry.toRoute()
ProfileScreen(profile)
}
}
For extra info, see this weblog publish.
CameraX Compose
We’re releasing a brand new Compose-specific CameraX artifact known as camera-viewfinder-compose. On this first alpha launch, you should use the brand new Viewfinder composable to show a digicam preview in your display that all the time exhibits the proper side ratio and rotation, whether or not you resize your window, unfold your machine, or change the show or orientation. It additionally accurately handles digicam and floor lifecycles, one thing that may be troublesome when coping with cameras. It additionally permits complicated interactions within the digicam coordinate system, making it simple so that you can implement gestures reminiscent of tap-to-focus or pinch-to-zoom.
You should utilize the Viewfinder composable even if you aren’t utilizing any of the opposite CameraX libraries, and hyperlink it on to your Camera2 code.
We’re actively engaged on enhancing the APIs and are trying ahead to your suggestions! Take a look at the recognized points, and elevate bugs or function requests to assist us create the absolute best digicam expertise in Compose.
Get began
We’re grateful for all the bug stories and have requests submitted to our challenge tracker that led to those adjustments — they assist us to enhance Compose and construct the APIs you want. Please proceed offering your suggestions, and assist us make Compose higher for you.
Questioning what’s subsequent? Take a look at our roadmap to see the options we’re presently fascinated by and dealing on. We are able to’t wait to see what you construct.
Pleased composing!
iQOO Z7 Pro 5G (Blue Lagoon, 8GB RAM, 256GB Storage) | 3D Curved AMOLED Display | 4nm MediaTek Dimesity 7200 5G Processor | 64MP Aura Light OIS Camera | Segment's Slimmest & Lightest Smartphone
₹24,999.00 (as of May 14, 2024 14:12 GMT +00:00 - More infoProduct prices and availability are accurate as of the date/time indicated and are subject to change. Any price and availability information displayed on [relevant Amazon Site(s), as applicable] at the time of purchase will apply to the purchase of this product.)Apple 20W USB-C Power Adapter (for iPhone, iPad & AirPods)
₹1,699.00 (as of May 14, 2024 14:12 GMT +00:00 - More infoProduct prices and availability are accurate as of the date/time indicated and are subject to change. Any price and availability information displayed on [relevant Amazon Site(s), as applicable] at the time of purchase will apply to the purchase of this product.)SAMSUNG EVO Plus 128GB Micro SDXC w/SD Adaptor, Up-to 160MB/s, Expanded Storage for Gaming Devices, Android Tablets and Smart Phones, Memory Card, MB-MC128SA/IN
₹1,109.00 (as of May 14, 2024 14:12 GMT +00:00 - More infoProduct prices and availability are accurate as of the date/time indicated and are subject to change. Any price and availability information displayed on [relevant Amazon Site(s), as applicable] at the time of purchase will apply to the purchase of this product.)OnePlus Nord Buds 2r True Wireless in Ear Earbuds with Mic, 12.4mm Drivers, Playback:Upto 38hr case,4-Mic Design, IP55 Rating [Deep Grey]
₹1,799.00 (as of May 14, 2024 14:12 GMT +00:00 - More infoProduct prices and availability are accurate as of the date/time indicated and are subject to change. Any price and availability information displayed on [relevant Amazon Site(s), as applicable] at the time of purchase will apply to the purchase of this product.)Portronics Luxcell Wireless Mini 10k 10000mAh 15W Magnetic Wireless Fast Charging Smallest Power Bank with 22.5 Wired Output Compatible with iPhone 12 & Above & Other QI Enabled Devices(Black)
₹1,469.00 (as of May 14, 2024 14:12 GMT +00:00 - More infoProduct prices and availability are accurate as of the date/time indicated and are subject to change. Any price and availability information displayed on [relevant Amazon Site(s), as applicable] at the time of purchase will apply to the purchase of this product.)Ambrane Unbreakable 3A Fast Charging 1.5m Braided Type C Cable for Smartphones, Tablets, Laptops & other Type C devices, 480Mbps Data Sync, Quick Charge 3.0 (RCT15A, Black)
₹129.00 (as of May 14, 2024 14:12 GMT +00:00 - More infoProduct prices and availability are accurate as of the date/time indicated and are subject to change. Any price and availability information displayed on [relevant Amazon Site(s), as applicable] at the time of purchase will apply to the purchase of this product.)Portronics My Buddy K Portable Laptop Stand with Adjustable Height, Foldable, OverHeating Protection for Laptops & MacBooks (Grey)
₹498.00 (as of May 14, 2024 14:12 GMT +00:00 - More infoProduct prices and availability are accurate as of the date/time indicated and are subject to change. Any price and availability information displayed on [relevant Amazon Site(s), as applicable] at the time of purchase will apply to the purchase of this product.)Rellon Industries Study Table for Students Bed Table for Study Foldable Laptop Table Portable & Lightweight Mini Table Bed Reading Table,Laptop Stands, Laptop Desk (A1)
₹599.00 (as of May 14, 2024 14:12 GMT +00:00 - More infoProduct prices and availability are accurate as of the date/time indicated and are subject to change. Any price and availability information displayed on [relevant Amazon Site(s), as applicable] at the time of purchase will apply to the purchase of this product.)Zebronics ZEB-KM2100 Multimedia USB Keyboard Comes with 114 Keys Including 12 Dedicated Multimedia Keys & with Rupee Key
₹199.00 (as of May 14, 2024 14:12 GMT +00:00 - More infoProduct prices and availability are accurate as of the date/time indicated and are subject to change. Any price and availability information displayed on [relevant Amazon Site(s), as applicable] at the time of purchase will apply to the purchase of this product.)Dyazo 6 Angles Adjustable Aluminum Ergonomic Foldable Portable Tabletop Laptop/Desktop Riser Stand Holder Compatible for MacBook, HP, Dell, Lenovo & All Other Notebook (Silver)
₹399.00 (as of May 14, 2024 14:12 GMT +00:00 - More infoProduct prices and availability are accurate as of the date/time indicated and are subject to change. Any price and availability information displayed on [relevant Amazon Site(s), as applicable] at the time of purchase will apply to the purchase of this product.)Auto Amazon Links: No products found.